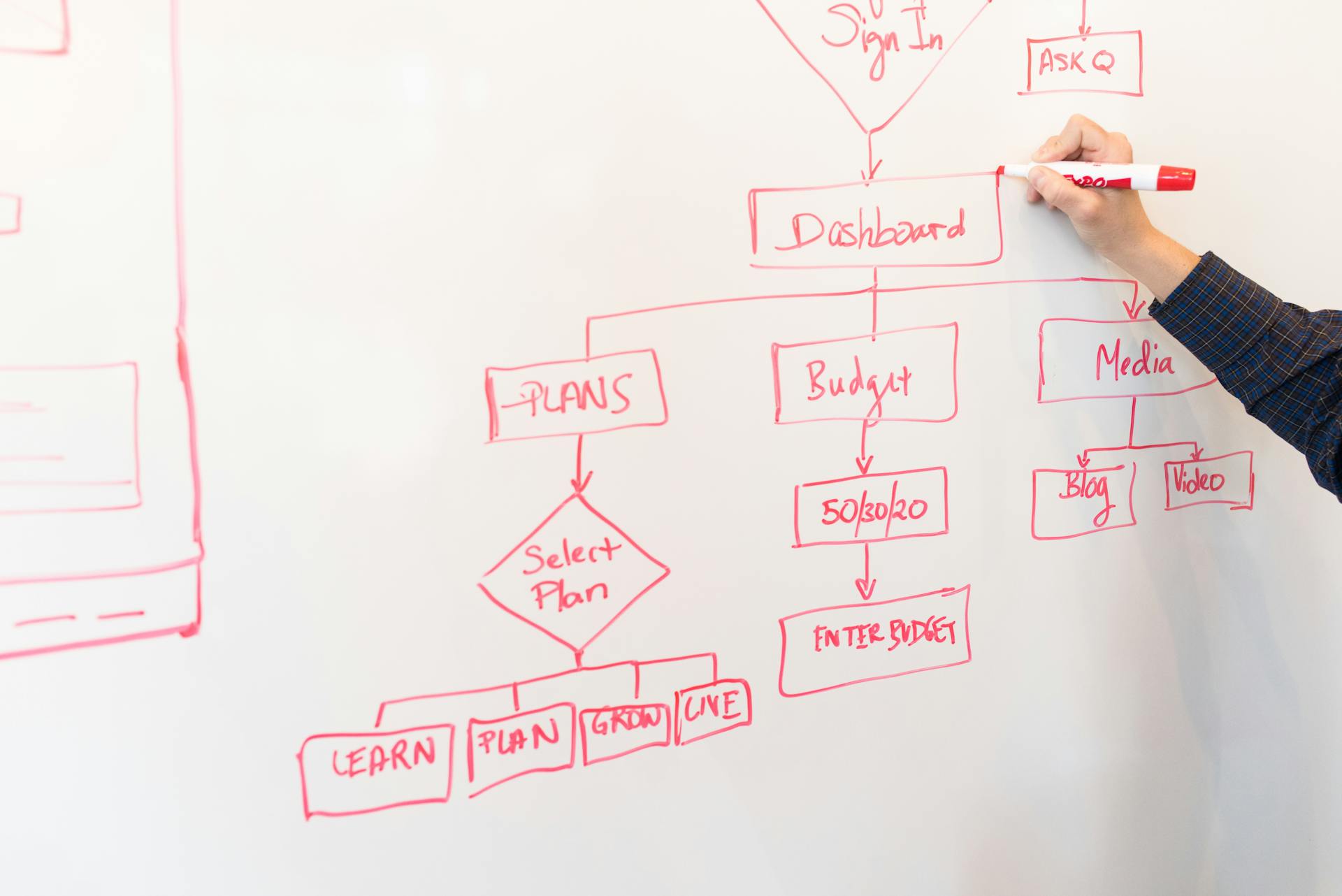
Grokking functional programming fundamentals is all about understanding how to write code that's composable, modular, and easy to reason about. This means breaking down complex problems into smaller, manageable pieces.
One key aspect of functional programming is immutability, which means that data should never be changed once it's created. As we saw in the example of the immutable list, this makes it easier to predict how the data will behave.
Immutability also helps to prevent side effects, which can make code harder to understand and debug. By avoiding side effects, we can write more predictable and reliable code.
In functional programming, functions are treated as first-class citizens, which means they can be passed around like any other value. This allows us to write more flexible and reusable code.
For your interest: Grokking Data Structures
The Basics
In functional programming, functions are the building blocks of code, and understanding their types is crucial. A function type signature indicates the types of inputs and outputs.
Functions can take multiple inputs, but in a simple example, a function takes a string as input and returns an int as output. The arrow (->) separates the input type from the output type.
In F#, the function type signature is used to define the expected input and output types. This helps catch errors early and makes the code more readable.
The arrow (->) is a key part of a function type signature, separating the input type from the output type. This notation is used consistently throughout functional programming.
Functions
Functions are a fundamental concept in functional programming, and they're used in a variety of ways. Implementing requirements as functions can make code more modular and easier to understand.
Function signatures should tell the whole story, providing all the necessary information about what a function does and what it returns. Using Java's Function values allows for more flexibility and expressiveness in programming.
Functions can be passed as arguments to other functions, and this is a powerful technique for reducing code duplication. Passing user-defined functions as arguments can make code more reusable and maintainable.
Four Functions
Functions can be incredibly powerful tools in programming, and one of the key aspects of functions is that they can be used as values.
Functions can be passed as arguments to other functions, which allows for a high degree of flexibility and modularity in our code. This is demonstrated in section 4.9, where it's shown that we can pass user-defined functions as arguments to other functions.
Functions can also be returned from other functions, which enables a whole new level of abstraction and reuse in our code. For example, section 4.37 shows that functions can return functions, and section 4.38 demonstrates how to use functions that can return functions.
Functions can even be used to reduce many values into one value, which is a key concept in functional programming. Section 4.52 shows how to use the foldLeft function to achieve this, and section 4.53 provides a deeper dive into the foldLeft function and its uses.
By using functions as values, we can write more concise and expressive code that is easier to understand and maintain. This is demonstrated in section 4.58, which shows how to use more concise syntax for inline functions.
9 Io
I'd love to dive into the world of Io functions. Io is a fascinating programming language that's all about functions, and one of the coolest things about it is how it handles function arguments.
In Io, functions can take any number of arguments, and they don't even need to be declared beforehand. This is because Io uses a concept called "call-by-value", which means that the function receives a copy of the argument's value, rather than the argument itself.
Functions in Io can also return multiple values, which is super useful for tasks like data processing and error handling. For example, a function might return a value and an error message, making it easier to handle errors in a program.
One thing that's really unique about Io functions is that they can be used as first-class objects. This means that functions can be passed around, stored in variables, and even returned from other functions. It's a powerful feature that makes Io code incredibly flexible and modular.
In Io, functions can also be defined recursively, which is a game-changer for tasks like tree traversal and data processing. By using recursion, you can write functions that are much more concise and easier to understand than their iterative counterparts.
The Io language also supports function closures, which are functions that capture their surrounding environment. This means that a closure can have access to variables and functions that were defined outside of it, making it a powerful tool for tasks like data processing and algorithm implementation.
Functions in Io can be combined using a concept called "function composition", which allows you to create new functions by chaining together existing ones. This is a great way to build complex functions from simpler ones, making it easier to write and maintain code.
Overall, Io functions are incredibly powerful and flexible, making it a great language for tasks that require a lot of code reuse and modularity.
Intriguing read: Learn How to Code Google's Go Programming Language
Sources
- https://www.gobookshop.com/product/9781617291838/
- https://www.compositional-it.com/news-blog/grokking-function-type-signatures/
- https://blackwells.co.uk/bookshop/product/Grokking-Functional-Programming-by-Michal-Plachta/9781617291838
- https://knjige.kombib.rs/grokking-functional-programming
- https://f3yourmind.net/2014/10/30/update-on-grokking-functional-programming/
Featured Images: pexels.com