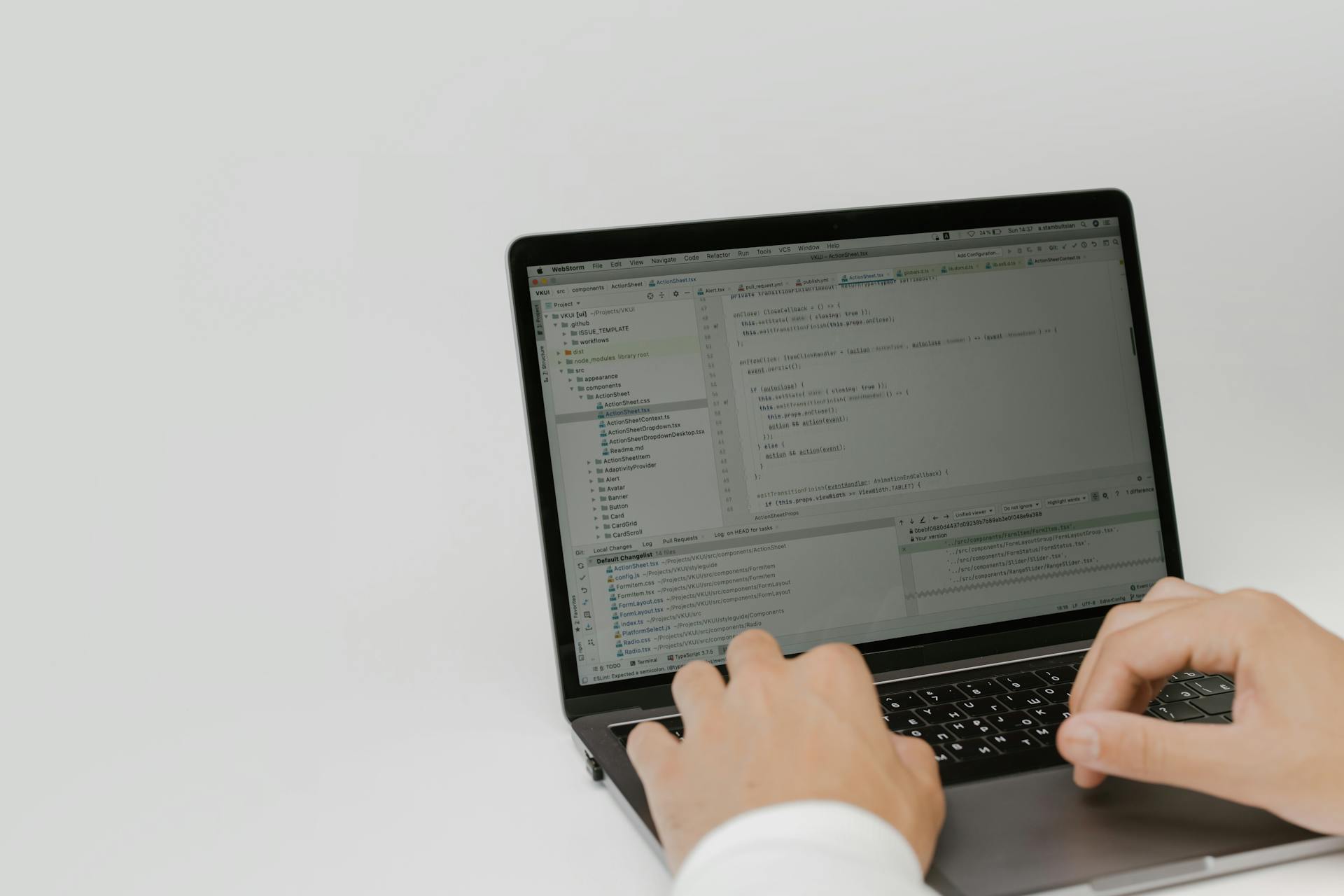
Coding a Tetris game in Python can be a fun and rewarding experience. You'll need to start by importing the necessary libraries, including Pygame for game development.
First, install Pygame using pip, the Python package manager. This will give you access to the functions and tools you need to create a game.
Next, initialize the Pygame library and set up the game window. This will establish the canvas where your game will be played out.
The game window will be 10 units wide and 20 units tall, with a title bar that displays the game's name.
Setting Up the Environment
To start coding your Tetris game in Python, you need to set up your development environment. This involves installing the necessary tools and software, choosing a code editor, and configuring a testing environment.
First, you need to have Python installed on your computer. Python is a versatile programming language widely used in game development due to its simplicity and power. Download Python from the official Python website and follow the installation instructions for your operating system.
Check this out: Formal Operational Stage of Development
To ensure Python is installed correctly, make sure to check the option to add Python to your system's PATH during installation.
Once Python is installed, you need to install the Pygame library. Pygame is a set of Python modules designed for writing video games, providing functionalities like creating windows, drawing shapes, handling input, and more.
To install Pygame, open a terminal or command prompt and run the following command: `pip install pygame`. This command will download and install the Pygame library, making it ready to use in your projects.
Here are the steps to install Python and Pygame:
- Download and install Python from the official Python website.
- Check the option to add Python to your system's PATH during installation.
- Open a terminal or command prompt.
- Run the command `pip install pygame` to install Pygame.
Creating the Window
Now that we have our development environment set up, let's create the game window. This is where the magic happens, and the game comes to life.
We'll start by defining the tetromino shapes, which are the building blocks of the game. These shapes will be used to create the game board and pieces that the player will interact with.
You might enjoy: Unity Learn Create with Code
The game window is essentially a container that holds the game board and other graphical elements. We'll define its properties, such as size and position, to ensure it fits perfectly on the screen.
In our development environment, we've already set up the necessary tools to create the game window. Now, it's time to put the code into action.
The game window will be created using a combination of code and graphical elements. We'll use a library or framework to handle the graphical aspects, making it easier to focus on the game logic.
Our game window will be a critical component of the game, providing a clear and intuitive interface for the player. We'll make sure it's visually appealing and easy to navigate.
Defining Screen Dimensions, Colors, and Tetromino Shapes
To get started with building our Tetris game, we need to define some constants for our game, such as screen dimensions, grid size, colors, and the shapes of the tetrominoes. We can define the width and height of the game window, the grid size for the tetrominoes, and the colors we will use for the game.
The SHAPES list contains the tetromino shapes represented as strings, and we're free to add or edit as we wish. We can also play around with the colors and grid size to see what works best for our game.
Here are the specific constants we need to define:
We can use these constants to set up our game window and grid, and start building our tetrominoes.
Implementing the Classes
Now that we have our game window and tetromino shapes defined, let's create the Tetromino and Tetris classes to manage the game logic. We'll use these classes to implement the game's core functionality.
The Tetromino class will encapsulate the properties and methods related to a tetromino, such as its shape and position. The Tetris class will manage the overall game logic, including the movement and rotation of the tetrominoes.
We can use the Tetromino class to implement the rotate method, which cycles through the different rotations of the tetromino, and the move method, which adjusts its horizontal position based on user input. This will bring the tetrominoes to life and make the game more engaging.
Planning the
Planning the Tetris Game is crucial to its success. To create an engaging game, we need to consider the key rules and mechanics. Tetris is a tile-matching puzzle game where the player must manipulate falling tetrominoes to form complete lines without gaps.
The game ends when the tetrominoes stack up to the top of the playing field. The game has five key rules and mechanics: tetrominoes fall automatically, the player can move tetrominoes left, right, rotate them, and drop them faster, line clearing is a crucial aspect of the game, the game over condition is triggered when new tetrominoes can no longer be placed at the top of the playing field, and scoring is based on the number of lines cleared simultaneously.
The grid is a 10x20 rectangular field, with each cell being either empty or filled with part of a tetromino. The grid is visualized as a matrix where each element represents a cell. The width of the grid is 10 cells and the height is 20 cells.
The Tetris grid can be represented using a 2D list (list of lists) where each element is an integer. A value of 0 represents an empty cell, and any other value represents a filled cell with part of a tetromino.
Here are the key components of the game:
- Grid: The playing field where tetrominoes fall.
- Tetrominoes: The shapes that fall into the grid.
- Game Controls: Player inputs to move and rotate tetrominoes.
- Scoring: A system to track and display the player’s score based on lines cleared.
- Game Over Conditions: Criteria to determine when the game ends.
Creating the Class
Creating the Class is a crucial step in implementing the classes for our Tetris game. This involves defining the properties and methods of the Tetromino and Tetris classes.
The Tetromino class will represent a single tetromino piece, with properties for its position, shape, color, and rotation. This class will be used to create and manage individual tetromino pieces.
The Tetris class, on the other hand, will handle the main game logic. It will have methods for creating a new piece, checking if a move is valid, clearing lines, locking a piece, updating the game state, and drawing the game.
To create the Tetromino class, we'll need to add properties for its position, shape, color, and rotation. The Tetris class will have methods for creating a new piece, checking if a move is valid, clearing lines, locking a piece, updating the game state, and drawing the game.
On a similar theme: Create Feature for Dataset Huggingface
Here's a breakdown of the properties and methods for each class:
The Tetromino class will have properties for its position, shape, color, and rotation, while the Tetris class will have methods for creating a new piece, checking if a move is valid, clearing lines, locking a piece, updating the game state, and drawing the game.
Drawing Text Helper Functions
Drawing text is a crucial part of creating an engaging game like Tetris.
When creating helper functions for drawing text, we need to pass in four arguments: the screen to draw on, the current score, the x-coordinate of the position, and the y-coordinate of the position.
In our Tetris game, we have two helper functions: one for drawing the score and another for drawing the "Game Over!" message. These functions use the Pygame surface to draw on, which is the screen.
The functions create a font object using pygame.font.Font(None, 36), which sets the font size to 36. This font object is then used to render the score text with the font.render() method.
Here's a summary of the arguments our helper functions take:
- screen: the Pygame surface to draw on.
- score: the current game score.
- x: the x-coordinate of the position where the score will be displayed.
- y: the y-coordinate of the position where the score will be displayed.
These helper functions are a great way to keep our code organized and make it easier to draw text in our game.
Sources
- https://thepythoncode.com/article/create-a-tetris-game-with-pygame-in-python
- https://medium.com/wiki-flood/python-tetris-game-build-drop-repeat-ddaf0ed6804c
- https://copyassignment.com/tetris-game-in-python-code/
- https://medium.com/@amit25173/python-pygame-tetris-tutorial-6db54de4d43d
- https://www.bomberbot.com/lessons/tetris-python-tutorial-using-pygame-a-deep-dive-for-aspiring-game-developers/
Featured Images: pexels.com