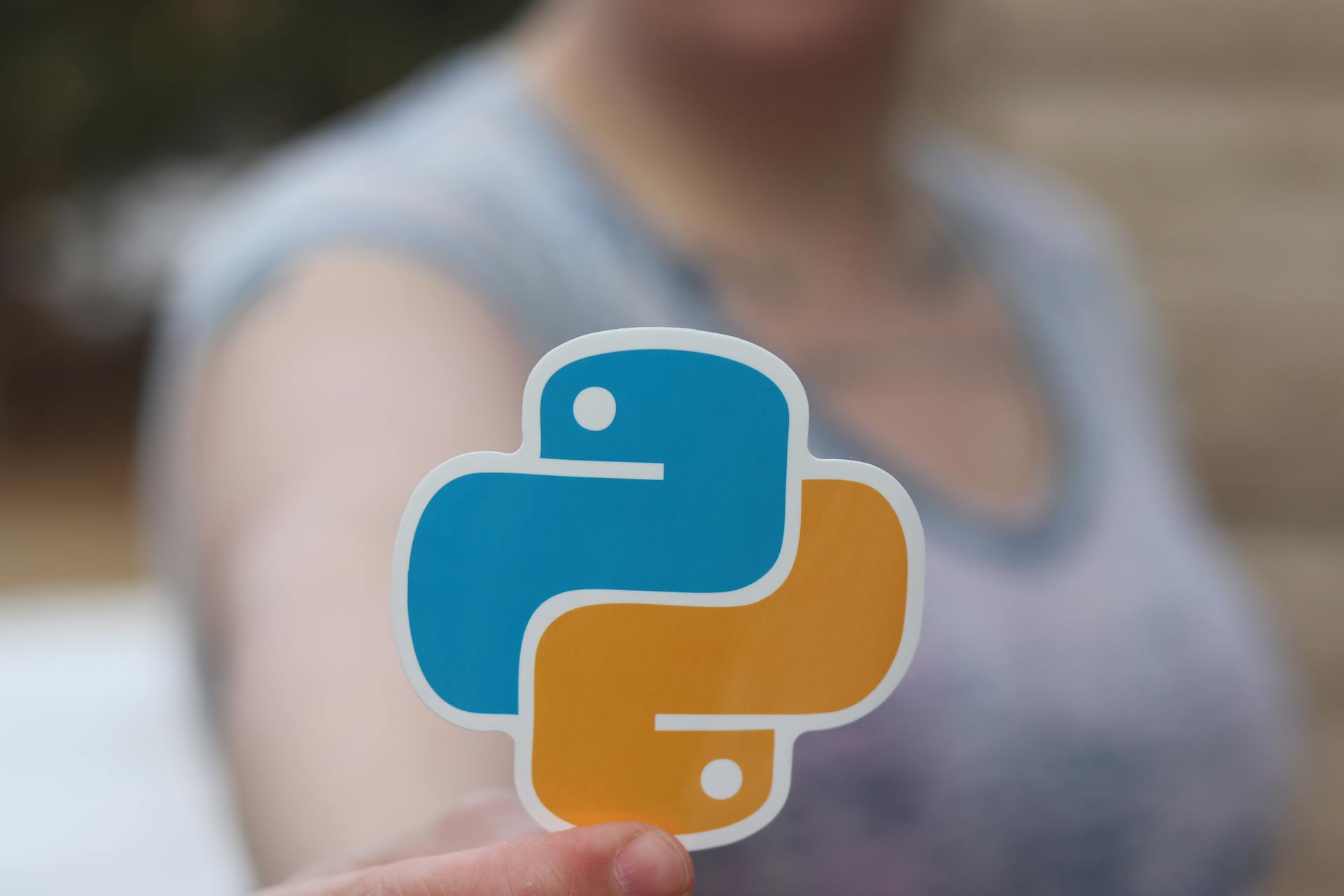
Learning to code Python is a game-changer, and for good reason - it's one of the most in-demand programming languages out there. With Python, you can create a wide range of applications, from simple scripts to complex data analysis tools.
Python is a versatile language that's easy to learn and use, making it perfect for beginners. Its syntax is simple and intuitive, and it has a vast collection of libraries and frameworks that make development a breeze.
As you start learning Python, you'll be able to automate tasks, work with data, and even build your own games. The possibilities are endless, and the best part is that you can start building projects right away.
Worth a look: Data Labeling in Machine Learning with Python Pdf
Getting Started
Getting Started with Python Programming is a great place to begin your coding journey. This section covers the essential elements you need to kickstart your Python programming skills.
To start, you'll need to learn the basics of Python, including syntax, keywords, and comments. Syntax is the set of rules that define the structure of your code, and it's what makes Python code readable and efficient.
Curious to learn more? Check out: Learn How to Code Google's Go Programming Language
Python has a vast collection of keywords that you'll use to perform various operations, such as conditional statements, loops, and functions.
Comments in Python are used to add notes to your code, making it easier for you and others to understand what your code is doing.
Variables in Python are used to store and manipulate data, and understanding how to use them is crucial to writing effective code.
Indentation is also important in Python, as it's used to define the structure of your code and make it more readable.
Here's a quick rundown of the key concepts you'll need to grasp:
- Learn Python Basics
- Syntax
- Keywords in Python
- Comments in Python
- Learn Python Variables
- Learn Python Data Types
- Indentation and why is it important in Python
Python Fundamentals
Python is a dynamic, interpreted language that stands out for its simplicity and versatility. It's a top choice for both beginners and professionals, and its syntax is clean and simple, making the code easy to understand and write.
Python's features include being easy to read and write, interpreted, object-oriented and functional, dynamically typed, and having extensive libraries. It's also cross-platform, meaning it can run on different operating systems like Windows, macOS, and Linux without modification.
Here are some key features of Python:
- Easy to Read and Write: Python’s syntax is clean and simple, making the code easy to understand and write, even for those new to programming.
- Interpreted Language: Python executes code line by line, which helps in easy debugging and testing during development.
- Dynamically Typed: You don’t need to specify data types when declaring variables; Python figures it out automatically.
- Extensive Libraries: Python has a rich collection of libraries for tasks like web development, data analysis, machine learning, and more.
- Cross-Platform: Python can run on different operating systems like Windows, macOS, and Linux without modification.
These features make Python an excellent choice for beginners and professionals alike.
Basics
Python is a dynamically typed language, meaning you don't need to specify data types when declaring variables; Python figures it out automatically.
This makes it easier to write code, but also requires attention to detail to avoid type-related errors. For example, if you assign a string to a variable, you can't suddenly use it as a number without causing an error.
Variable names are crucial in Python, as they help you (and others) understand what's going on in your code. Use meaningful names like "name" for a single name and "names" for a list of names. Python prefers underscored_parts for variable names made up of multiple words, but guides developers to defer to camelCasing if integrating into existing code.
Here are some key characteristics of Python:
- Easy to read and write
- Interpreted language
- Object-oriented and functional
- Dynamically typed
- Extensive libraries
- Cross-platform
- Community support
These features make Python a top choice for both beginners and professionals.
Data Types
Python's data types are incredibly versatile, allowing you to work with a wide range of data types, from strings to numbers to lists.
Strings are a fundamental data type in Python, and they can be used to store and manipulate text. Whether you're working with names, sentences, or entire paragraphs, strings are the way to go.
Numbers are another essential data type, and Python supports both integers and floating-point numbers. You can use them to perform calculations, store numerical values, and even generate random numbers.
Booleans are a special type of data that can only have two values: True or False. They're perfect for making decisions in your code, like checking if a user is logged in or not.
Python lists are a type of collection that allows you to store multiple values in a single variable. They're ordered, meaning you can access elements by their index, and they're mutable, so you can add or remove elements as needed.
Here's an interesting read: Data Labeling in Machine Learning with Python
Here's a quick rundown of the different data types you can work with in Python:
Type casting is also an important concept in Python, allowing you to convert one data type to another. This can be useful when working with different data types or when you need to perform specific operations.
The Language Reference
The Language Reference is a vital resource for any Python programmer. It's the official reference manual that covers the syntax and core semantics of the language.
You can find the Python Language Reference in the official Python documentation. It's a comprehensive guide that's perfect for beginners and experts alike.
The Language Reference is a must-read for anyone who wants to master Python. It's concise and to the point, covering everything from basic syntax to advanced language features.
Here are some key topics covered in the Language Reference:
- Arithmetic operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Membership & Identity Operators | Python "in", and "is" operator
These operators are essential for performing various tasks in Python, from simple arithmetic to complex logical operations.
The Language Reference is also a great resource for learning about Python's core language features, such as the "in" and "is" operators. These operators are used for testing membership in collections and comparing object identities.
The Python Essential Reference is another excellent resource that's written by David Beazley. It's a concise guide that covers both the core language and the standard library.
Intriguing read: What Is the Hardest Code Language to Learn
Scientific Primer
Dataquest offers an interactive way to learn Python and data science by analyzing interesting datasets, such as CIA documents and NBA player stats, and building complex algorithms like neural networks and decision trees.
If you're looking for more resources, the CS109 Harvard class has some of its projects and materials online, which can be a great way to learn from real-world examples.
You can also check out Scikit-learn Documentation, which has some great tutorials and documentation to help you get started with machine learning in Python.
For a more in-depth look at Python's usage in the scientific field, you might want to check out A Primer on Scientific Programming with Python, which covers examples from mathematics and the natural sciences.
This book is a great resource for those looking to learn Python for scientific programming, and it's a good starting point for anyone interested in using Python for data analysis and machine learning.
Functions and OOPs
Functions and OOPs are two fundamental concepts in Python programming. Python Functions are the backbone of organized and efficient code, with syntax, parameter handling, return values, and variable scope being key aspects to understand.
To write functions in Python, you'll need to grasp concepts like Python Function Global and Local Scope Variables, Return statements, and the use of the pass statement. You'll also learn about versatile functions like range() and powerful tools like *args and **kwargs for flexible parameter handling.
Here are some key concepts to get you started:
- Python Function Global and Local Scope Variables
- Use of pass Statement in Function
- Return statement in Python Function
- Python range() function
- *args and **kwargs in Python Function
Additionally, you'll learn about Object-Oriented Programming (OOP) concepts in Python, including classes, objects, polymorphism, inheritance, encapsulation, and iterators. These concepts will empower you to build modular, reusable, and scalable code.
Problem Solving with Algorithms
Python's conditional statements are pivotal in programming, enabling dynamic decision-making and code branching.
To write clear, efficient code that responds intelligently to various scenarios, you'll need to master Python's conditional logic, from basic if...else statements to nested conditions and the concise ternary operator.
One of the most common conditional statements in Python is the if-else statement, which allows you to execute different blocks of code based on a condition.
Python's if-elif-else ladder is another powerful tool for making decisions in your code, allowing you to check multiple conditions and execute different blocks of code accordingly.
Indentation is also a crucial aspect of Python programming, as it determines the flow of your code. According to the official Python style guide (PEP 8), you should indent with 4 spaces.
Here are some key concepts to keep in mind when problem-solving with algorithms:
- Conditional statements: if-else, if-elif-else, ternary operator
- Loop constructs: for loops, while loops, list comprehension, dictionary comprehension
- Indentation: 4 spaces, avoid using TABs
OOPs Concepts
Python's Object-Oriented Programming (OOP) concepts are the backbone of building modular, reusable, and scalable code. These concepts include encapsulation, inheritance, polymorphism, abstract classes, and iterators.
Encapsulation is the practice of hiding an object's internal state and behavior, making it harder for other parts of the code to access or modify it directly. This is achieved through the use of classes and objects in Python.
A class is essentially a blueprint for creating objects, and objects are instances of classes. In Python, you can define a class using the class keyword, followed by the name of the class and a colon.
Here's a quick rundown of the OOP concepts covered in Python:
- Python Classes and Objects
- Polymorphism
- Inheritance
- Abstract
- Encapsulation
- Iterators
Polymorphism allows objects of different classes to be treated as if they were of the same class, thanks to the use of inheritance and abstract classes. Abstract classes, on the other hand, provide a way to define a blueprint for objects that can be used as a base class for other classes.
Iterators are used to traverse sequences, such as lists or strings, and return the next item in the sequence each time you call the iterator.
Functions
Functions are the backbone of organized and efficient code in Python, allowing you to write reusable blocks of code that can be easily called upon when needed.
A function is defined using the def keyword, with its parameters within parentheses and its code indented. The first line of a function can be a documentation string, or docstring, that describes what the function does.
The Python Function Global and Local Scope Variables concept is crucial to understand, as it determines the accessibility of variables within a function. This is different from variables defined in other functions, which are separate and do not interfere with each other.
The pass statement in a function is used when a statement is required syntactically, but no execution of code is necessary. This is a common use case when defining a function but not implementing any code yet.
The return statement in a function can take an argument, in which case that is the value returned to the caller. This is useful for functions that need to return a value to the calling code.
Python's range() function is a versatile tool that generates a sequence of numbers, allowing you to iterate over a range of values. This is particularly useful in loops and for loops.
The *args and **kwargs in Python Function concept allows for flexible parameter handling, enabling you to pass a variable number of arguments to a function. This is achieved through the use of asterisks (*) and double asterisks (**).
Here's a list of some key concepts related to functions in Python:
- Python Function Global and Local Scope Variables
- Use of pass Statement in Function
- Return statement in Python Function
- Python range() function
- *args and **kwargs in Python Function
- Python closures
- Python ‘Self’ as Default Argument
- Decorators in Python
- Map Function
- Filter Function
- Reduce Function
- Lambda Function
Exception Handling
Exception handling is a crucial aspect of writing robust and fault-tolerant code. Python deals with unexpected errors using try and except blocks.
You can use try and except blocks to handle exceptions in Python. For example, you can use the try block to execute a code snippet and the except block to handle any exceptions that may occur.
Exception handling is not just about catching errors, it's also about understanding the type of error that occurred. Python has a range of built-in exception types, including ValueError, TypeError, and FileNotFoundError.
Here are some common built-in exception types in Python:
You can also define your own custom exception types in Python. This can be useful for creating domain-specific exceptions that are more meaningful to your application.
Hacking Secret Ciphers
Hacking Secret Ciphers is a great way to learn about cryptography and programming with Python. This book is perfect for absolute beginners, teaching them Python programming and basic cryptography.
The book covers various ciphers, providing source code for each one. It also includes programs that can break these ciphers, which is a great way to learn about cryptography.
This book is an excellent beginner programmer's guide to Python, covering topics from "hello world" in the console to web development.
You might like: Learn How to Code Books
Cookbook
The Cookbook is a treasure trove of practical recipes for Python developers. It's written by David Beazley and Brian K. Jones, who pack it with hands-on examples to help you master the core language and tackle common tasks.
This opinionated guide is a best practice handbook that covers installation, configuration, and usage of Python on a daily basis. It's geared towards both novice and expert developers, so whether you're just starting out or looking to refine your skills, you'll find something useful here.
One of the strengths of the Cookbook is its focus on real-world applications. By covering a wide range of tasks and domains, you'll be able to adapt your skills to any project that comes your way. From basic programming concepts to advanced topics like decorators and closures, the Cookbook has got you covered.
The Cookbook is an excellent resource to have in your toolkit, whether you're working on a personal project or collaborating with others on a team. Its practical approach and extensive coverage make it an invaluable asset for any Python developer.
Magic Methods Guide
Magic methods are surrounded by double underscores and can make classes and objects behave in different and magical ways.
Rafe Kettler's blog posts, found on his GitHub repository, explain these magic methods in detail. You can access the PDF version of his guide there.
Magic methods are used to make classes and objects behave in different and magical ways, and they're surrounded by double underscores.
Rafe Kettler's guide is a great resource for learning about magic methods, and it's available on his GitHub repository.
Curious to learn more? Check out: Is Transfer Learning Different than Deep Learning
Input/Output and Data
Learning to handle input and output in Python is a crucial part of coding. You can start by mastering the versatile print() function, which allows you to print messages to the screen.
The print() function has several parameters, including end and sep, that can be used to customize the output. For example, you can use the end parameter to print multiple lines without a newline character.
To receive user input, you can use the input() function, which allows you to take input from the user and store it in a variable. You can also take multiple inputs from the user by using the input() function in a loop.
Here's a summary of the key concepts:
- Python print() function
- Python | end parameter in print()
- Python | sep parameter in print()
- Taking Input in Python
- Taking Multiple Inputs from users in Python
By understanding these concepts, you'll be able to handle input and output in Python with ease, and start building more complex programs.
Packages
Python has a vast collection of packages that make it incredibly versatile.
Built-in modules in Python are a great place to start, providing a solid foundation for any project. These modules are included with the Python installation and offer a range of useful functions and classes.
Python Data Structures and Algorithm (DSA) libraries are another essential tool for any developer. They provide pre-built data structures and algorithms that can be used to solve complex problems.
Machine learning is a key application of Python, with libraries like scikit-learn and TensorFlow making it easy to build and train models.
Python GUI libraries, such as Tkinter and PyQt, allow developers to create visually appealing and user-friendly interfaces.
Web scraping packages like BeautifulSoup and Scrapy make it easy to extract data from websites.
Game development packages, including Pygame and Panda3D, enable developers to create engaging and interactive games.
Some popular web frameworks, including Django and Flask, provide a solid foundation for building web applications.
Image processing libraries like OpenCV and Pillow make it easy to manipulate and analyze images.
Here are some examples of Python packages and libraries:
- Built-in Modules
- Python DSA Libraries
- Machine Learning
- Python GUI Libraries
- Web Scraping Packages
- Game Development Packages
- Web Frameworks
- Image processing Libraries
Input/Output
Input/Output is a fundamental aspect of working with data in Python. It's essential for interacting with users and processing data effectively.
The print() function is a versatile tool in Python, allowing you to output text to the screen. You can use it to print variables, strings, and even complex data structures.
One of the most useful features of the print() function is its ability to handle formatting with the f-string. This allows you to embed expressions inside string literals, making it easier to create formatted output.
If you need to print without a newline, you can use the end parameter in the print() function. This is useful when you want to print multiple lines of text without adding an extra newline at the end.
The sep parameter in the print() function is another useful feature. It allows you to specify a separator to use between multiple arguments when printing.
Here are some common uses of the print() function:
- Printing variables: `print(my_variable)`
- Printing strings: `print("Hello, world!")`
- Printing complex data structures: `print(my_list)`
Taking user input is also a crucial aspect of input/output in Python. You can use the input() function to receive user input, which returns a string.
If you need to take multiple inputs from users, you can use the split() method to split the input string into individual inputs.
Database Handling
Database handling is a crucial aspect of working with data. Python provides robust support for accessing and working with different types of databases.
You can access and work with MySQL databases using Python. Python also allows you to work with MongoDB databases.
To work with MySQL databases, you'll need to learn how to access and work with them. This includes understanding how to query and manipulate data in a MySQL database.
In Python, you can use various libraries and tools to interact with MongoDB databases. This includes learning how to perform CRUD (Create, Read, Update, Delete) operations on MongoDB data.
Python's database handling capabilities make it a popular choice for data-driven applications. Whether you're working with MySQL or MongoDB, Python provides a flexible and powerful way to manage your data.
Frequently Asked Questions
Is Python code easy to learn?
Yes, Python is considered one of the easiest programming languages for beginners to learn. Its simplicity makes it a great starting point for those new to coding.
How to learn coding in Python?
To learn coding in Python, start by identifying your motivation and learning the basic syntax, then progress to structured projects and eventually work on challenging projects on your own. Follow these steps to become proficient in Python programming.
Featured Images: pexels.com