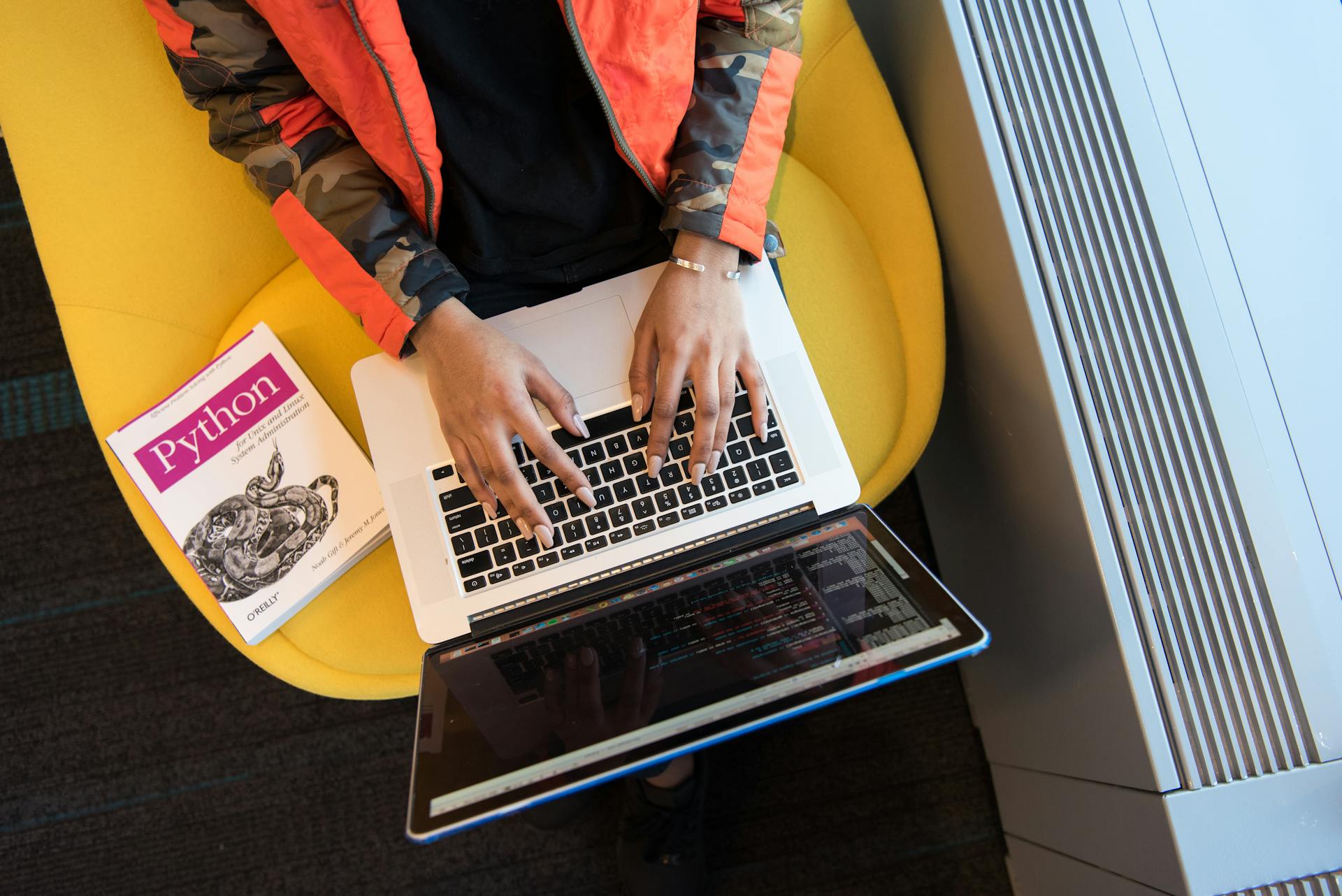
Learning to code can be a daunting task, but having the right skills can make all the difference. Understanding data structures and algorithms is crucial for any developer, as they form the building blocks of software development.
Data structures like arrays and linked lists are fundamental concepts that every developer should know. In our book, we explore these data structures in-depth, providing practical examples and exercises to help you master them.
Algorithms, on the other hand, are the instructions that tell a computer what to do with the data. Understanding how to design and implement efficient algorithms is essential for writing scalable and maintainable code.
Why Learn to Code
Learning to code is a valuable skill that can open doors to new opportunities. It builds logical thinking and problem-solving skills, making you a more versatile and resourceful individual.
Learning coding from scratch lays a solid technical foundation to advance upon. This foundation enables you to adapt more readily to new languages.
One of the biggest advantages of learning to code is the flexibility it offers. With coding skills, you can customize software to meet your own needs, giving you control and autonomy.
Learning to code can also lead to lucrative tech careers with lots of flexibility. It's a skill that's in high demand and can be applied across various industries.
Here are some of the main advantages of starting from the basics:
- Builds logical thinking and problem-solving skills
- Lays a solid technical foundation to advance upon
- Allows you to customize software to meet your own needs
- Opens doors to lucrative tech careers with lots of flexibility
Getting Started
Start small and focus on learning language basics before tackling big projects.
To begin, define what you want to achieve. Do you want to build websites, mobile apps, games? Identify a coding language to focus on first like Python, JavaScript, etc. Setting goals will help guide your learning path.
Setting a broad goal like learning to code can be overwhelming, so break it down into smaller, more specific goals. For example, say you want to build a mobile app for your friend who is training for a half-marathon one year from now.
You might enjoy: Learn How to Code Google's Go Programming Language
Here are some key steps to help you start learning coding from scratch:
- Set realistic goals based on your skills, interests, and time commitments
- Choose a beginner-friendly programming language like Python, JavaScript, or Ruby
- Practice coding daily through tutorials, sample projects, and coding challenges
- Don't get discouraged - learning to code takes time and dedication
You can also leverage free online coding courses like Codecademy, freeCodeCamp, and W3Schools, and join online developer communities like GitHub, Stack Overflow, and Reddit to connect with other coders.
Intriguing read: Learn Morse Code Online
Programming Fundamentals
Learning to code takes patience, practice, and persistence. But by following these key steps, you'll establish critical foundations for programming success as a beginner.
To start, you should ask yourself why you want to learn to code. This reflection will help guide your learning journey and give you direction. Understanding your goals and aspirations is crucial to choosing the right coding tools, software, and programming language to get started.
Here are the 8 steps to learn coding fundamentals:
- Step 1: Ask Yourself, “Why Should I Learn to Code?”
- Step 2: Choose the Right Coding Tools and Software to Get Started
- Step 3: Pick the Right Programming Language to Start Learning
- Step 4: Start Your First Coding Project
- Step 5: Write Comments in Your Code
Mastering control structures, such as conditional statements and loops, is key to directing how programs execute and dynamically responding to different conditions.
Variables and Data Types
Variables and Data Types are the foundation of any program, and understanding them is crucial to storing, accessing, and modifying data.
A variable acts as a container for information that can be referenced and updated in a program. You can think of it as a labeled box where you store a value.
You give each variable a custom name to represent what kind of data it holds, like "userName" or "age". This makes it easy to reference and work with the data later.
Variables have an associated data type that determines what type of data they can store, like numbers, text, or true/false values. Common data types include:
To work with a variable's data, you simply reference it by name. For example, you'd write "userName = "Mary"" to store the text "Mary" in the "userName" variable. This is a fundamental concept in programming, and it's essential to understand it to write efficient and effective code.
Control Structures
Control Structures are the backbone of programming, allowing you to direct the flow of your code and make decisions based on conditions. This is crucial for creating dynamic and responsive programs.
Control Structures give you the power to conditionally execute code or repeat blocks of code, making them a fundamental concept in programming. Mastering control structures is key to directing how programs execute and dynamically responding to different conditions.
There are two most common control structures: Conditional statements and Loops. Conditional statements allow you to check logical conditions and execute different code blocks based on the outcome. Loops, on the other hand, allow you to repeat a block of code multiple times.
Here are some examples of how control structures can be used:
- Conditional statements: If-else statements, switch statements, etc.
- Loops: For loops, while loops, do-while loops, etc.
Control structures can be used to create complex logic and decision-making processes in your code. By mastering control structures, you can create programs that are more efficient, responsive, and user-friendly.
In programming, the order in which statements execute in a program is called control flow. This is where control structures come into play, allowing you to manipulate the flow of your code and create dynamic programs.
Worth a look: Create with Code Unity Learn
Functions
Functions are a fundamental concept in programming that help manage complexity and make code more maintainable.
A function is a reusable block of code that can be called from multiple places in a program, promoting modular code.
Functions help you break down complex tasks into smaller, named steps, which improves code readability by giving names to these steps.
Encapsulating complex logic in a function hides those details, making your code more organized and easier to understand.
Functions allow you to "DRY" your code, meaning you don't repeat yourself, which reduces errors and makes maintenance easier.
Here are the benefits of using functions:
- DRY code - Don't Repeat Yourself
- Reusability - Functions can be called anywhere
- Readability - Gives names to steps
- Encapsulation - Hides complex details
Functions become critical as programs get more advanced, helping you manage complexity and write more efficient code.
SQL in 10 Minutes
If you're looking to learn SQL quickly, there's a great book called SQL in 10 Minutes, Sams Teach Yourself. It's a short-and-sweet coding book with 22 ten-minute lessons.
This book is ideal for those who want to complete tasks in SQL quickly and methodically. It covers basic data retrieval and more in-depth topics like subqueries, table constraints, etc.
Mastering Advanced Techniques
To take your programming skills to the next level, you need to focus on advanced concepts like data structures, algorithms, design patterns, and architecture principles.
The book "Mastering Advanced Coding Techniques" recommends picking a specific language or framework and aiming to master it completely. This will help you become more efficient and confident in your coding abilities.
Data structures, such as those covered in "Computer Science Distilled", are essential for any programmer to learn. They will help you solve complex problems and improve your code's performance.
Algorithms are another crucial aspect of advanced programming. "Mastering Advanced Coding Techniques" suggests working through coding challenges on platforms like LeetCode and HackerRank to improve your algorithmic skills.
To write better code, you need to learn techniques like debugging, profiling, and optimization. "Mastering Advanced Coding Techniques" offers guidance on how to do this.
Here are some key areas to focus on when mastering advanced techniques:
By mastering these advanced techniques, you'll be able to tackle complex projects and take your programming skills to the next level.
Refactoring: Improving Existing Code
Refactoring is a crucial aspect of programming that involves improving poorly designed, inefficient programs to make them simpler and easier to maintain. This process can be a game-changer for large legacy code bases.
Refactoring is not just about cleaning up code, it's about making it more efficient and easier to work with. The book "Refactoring: Improving the Design of Existing Code" breaks down the refactoring techniques you need to know to achieve this.
Refactoring can be a daunting task, especially when working with messy code. However, with the right approach and techniques, you can transform your code into a well-designed, maintainable system.
The book "Refactoring: Improving the Design of Existing Code" starts with a "messy" sample application and guides you through the process of refactoring it to make it as efficient as possible. This is a great way to learn refactoring techniques and see the process in action.
By applying refactoring techniques, you can simplify your code and make it more efficient, which can save you time and reduce errors.
Web Development
Learning web development is a fantastic way to build a career in tech. HTML and CSS are the building blocks of web development, and learning them early on allows you to understand the structure and styling of web pages.
You can quickly build basic websites as you progress to other languages like JavaScript. HTML provides the content structure, while CSS handles the visual styling. Many coding apps and courses use projects like designing websites to teach programming basics to beginners.
To become a full stack developer, consider expanding beyond front-end or back-end. This allows you to understand the entire architecture of a web application and learn skills like database design, server configuration, and API development.
Here are some small, manageable projects to get you started:
- Build a personal website or blog using HTML, CSS, and JavaScript.
- Create simple games like tic-tac-toe or a text adventure game with Python or JavaScript.
- Build web or mobile apps that solve real problems.
- Start with projects that excite you!
Benefits of Projects
Projects are a must for entry-level programming jobs, as they prove competency in a given language. They show that you can build something from scratch, which is a valuable skill to have.
Projects keep you motivated by helping solidify the "why" behind your coding and setting clear, tangible benchmarks for your progress. Each completed project means one more skill under your belt.
Coding projects give you something to show for your work, which is a big plus when applying for jobs. It's one thing to put a language on your resume, but it's another to show you built an entire website or application from scratch.
Here are some classic projects to get you started:
- A time converter, in which the user submits a number of seconds, and your program gives the equivalent in hours, minutes, days, etc.
- A random number generator, which produces a random number between two values specified by the user.
- A calculator, in which the user specifies their inputs and mathematical operation, and your program gives an output.
- An address book, in which users can submit contact names, then search for contacts stored in your program.
- An alphabetizer, in which the user offers a list of words, and your program sorts them in alphabetical order.
- A hangman game, in which the user tries to guess a hidden word by inputting letters, and your game gives feedback for correct or incorrect guesses.
HTML and CSS: Web Development Building Blocks
HTML and CSS are the building blocks of web development, and learning them early on can give you a solid foundation for creating web pages. HTML provides the content structure, while CSS handles the visual styling.
You can quickly build basic websites as you progress to other languages like JavaScript. Many coding apps and courses use projects like designing websites to teach programming basics to beginners.
Curious to learn more? Check out: Learn to Code Html and Css Develop and Style Websites
With HTML and CSS working hand-in-hand, you'll be able to create visually appealing web pages. This combination is essential for web presentation.
Here are some benefits of learning HTML and CSS:
- Understand the structure and styling of web pages
- Quickly build basic websites as you progress to other languages like JavaScript
- Gain a solid foundation before diving into full stack development
For beginners, learning HTML and CSS can be made easy with the right resources. Books like "HTML and CSS: Design and Build Websites" by Jon Duckett offer illustrations, photography, and an accessible writing style to help you learn.
If you're looking for free coding resources, W3Schools is a comprehensive online resource that offers beginner-friendly documentation and tutorials for languages like HTML, CSS, and JavaScript.
Android App Development for Dummies
Android app development is a crucial aspect of web development, and thankfully, there are resources available to help you get started.
You can find comprehensive guides like "Android Application Development All-in-One For Dummies" that cover key programming concepts to program phone features and build and refine your apps.
This guide will also teach you how to manage data and use the Android native development kit.
The "Head First Android Development: A Brain-Friendly Guide" is another great resource that uses visual illustrations to keep you engaged and learn quickly.
You can use this guide to build a working app and improve it with interactive design and cross-device functionality.
On a similar theme: Learn Morse Code App
Android Development: Brain-Friendly
Coding is a skill that empowers you to create, innovate, and solve problems in new ways. It's a must-have for anyone looking to make a career pivot into tech or switch to a more technical role within their field.
You can start developing Android apps with comprehensive guides like "Android Application Development All-in-One For Dummies" that cover key programming concepts.
This guide will help you program phone features, build and refine your apps, manage data, and use the Android native development kit.
A brain-friendly guide like "Head First Android Development: A Brain-Friendly Guide" can also help you quickly build a working app and improve it with interactive design, cross-device functionality, and more.
Coding knowledge is an asset even if you're not pursuing a strictly technical role, showing technical know-how, the ability to grasp abstract concepts, and that you can solve complex problems.
Knowing at least one relevant programming language is a must, and for Android app development, learning Kotlin is a great starting point, with a complete introduction to coding in Kotlin found in "Head First Kotlin: A Brain-Friendly Guide".
Broaden your view: Learn to Code an App
Programming Languages
Learning to code can be overwhelming, but it doesn't have to be. There are hundreds of programming languages out there, each with its own unique purpose and capabilities.
Programming languages give you a structure for the instructions you're writing, and they look like English, but not exactly. Programmers call the terms and grammar in a programming language syntax.
Low-level languages like Assembly or Machine are easier for machines to read than humans, making them tough to learn. But don't worry, you can start with a middle-level language like C++ if you want to write operating systems, database systems, or image or video processing software.
Here are some of the best languages for beginners:
- HTML and CSS for web development
- C++ for writing operating systems, database systems, or image or video processing software
JavaScript: A Versatile Programming Language
JavaScript is a versatile programming language that runs practically everywhere, especially in web browsers. It's a must-learn for aspiring coders, and its prevalence in browser-based coding apps makes it readily accessible for beginners.
JavaScript can be used for a wide range of tasks, including adding dynamic interactivity to websites, building full stack web apps, and even mobile development, databases, and servers. With JavaScript, you can turn static web pages into dynamic ones, enabling page elements to move and react to user actions.
Take a look at this: Learn to Code Js
One of the best ways to learn JavaScript is through hands-on experience, and there are many excellent books available for beginners. For example, "JavaScript: Top Rated JavaScript Books" recommends two top-rated books for beginners, while "JavaScript: A Playful Introduction to Programming" offers a fun and relaxed introduction to the language.
If you're interested in mastering JavaScript, focus on DOM manipulation and asynchronous programming to bring your web pages to life. With practice and dedication, you can become proficient in JavaScript and unlock its full potential.
Here are some key benefits of learning JavaScript:
- High-level language that reads similarly to English
- Lots of built-in functionality so you can focus less on nitty-gritty details
- Huge community support for beginners with extensive tutorials and documentation
- Versatile for uses like data analysis, machine learning, web backends, and more
Kotlin Cookbook
Kotlin Cookbook is a problem-focused approach that can help you learn how to solve problems with Kotlin. This book is suitable for both experienced programmers and those new to Kotlin.
It includes over 80 code examples paired with simple explanations. This makes it easy to understand and apply Kotlin concepts in your own projects.
The book concentrates on your own use cases rather than on basic syntax, which is a great way to learn by doing. This approach can help you become a proficient Kotlin programmer in no time.
You can find this book on Amazon, which is a great resource for learning programming languages.
On a similar theme: Can I Learn to Code on My Own
Rust: Fast and Safe Systems Development
Rust is a multi-paradigm, general-purpose programming language designed for performance and safety.
Rust has a unique set of features that make it an attractive choice for systems development. It's fast and efficient, allowing developers to create high-performance systems.
One of the key benefits of Rust is its focus on safety. It helps prevent common errors like null pointer dereferences and data corruption. This makes it a great choice for building systems where reliability is crucial.
Rust's ownership and borrowing system is a fundamental concept in the language. It helps developers manage memory and prevent common errors like memory leaks.
Rust also has traits and generics, which allow developers to write flexible and efficient code. This makes it easier to write code that can work with different data types and scenarios.
Rust's core concepts are designed to be easy to learn and use. With the right resources, developers can quickly get up to speed with the language and start building high-performance systems.
See what others are reading: Learn to Code Rust
Objective-C Resources
Objective-C Resources can be a bit overwhelming, especially for beginners. There are some amazing books out there that can help you improve your skills.
One such book is "Objective-C Programming: The Big Nerd Ranch Guide" by Aaron Hillegass and Mikey Ward. This book is based on the popular Big Nerd Ranch Bootcamp and has an engaging style that makes learning fun.
It's divided into 52 sets of scenarios, tips, and shortcuts that will help you master Objective-C in no time. This coding book is concise and practical, covering C, Objective-C, and Apple technologies.
This book is a must-read for anyone looking to improve their Objective-C skills. It will help you avoid little-known pitfalls and choose the best, most efficient option possible.
For more insights, see: Books to Help Learn Code in Java
iOS Swift Mastery
To become an iOS pro, you'll need to dive into the world of Swift programming. This is the language of Apple, and mastering it will open doors to developing amazing apps.
Swift is an amazing language, and there are many resources available to help you learn it effectively. The Big Nerd Ranch Guide is an excellent starting point, covering the hows and whys of Swift, its grammar and style, and providing you with the knowledge and confidence to tackle coding challenges.
To take your iOS skills to the next level, you'll need to practice building apps. The Big Nerd Ranch Guide offers a comprehensive approach to iOS development, teaching you the foundations and tools you need to create apps with awesome features.
If you're looking for a more hands-on approach, consider the capstone projects in the Go coding book. These projects feature spacefaring gophers, Mars rovers, ciphers, and simulations, and are designed to help you learn concepts through practical application.
However, if you're focused on iOS development, the Big Nerd Ranch Guide is still an excellent choice. It's a concise and practical book that covers C, Objective-C, and tips for working with Apple technologies.
Curious to learn more? Check out: Learn to Code Swift
Books and Guides
If you're a visual learner, you'll love "Head First Python: A Brain-Friendly Guide". This book is designed to help you grasp Python concepts quickly, and you'll even get to build your own web application.
"Head First Python" has 52 exercises that drive concepts home, making it one of those programming textbooks you can actually work on, not just sit and read through.
Related reading: Learn to Code in Python Free
Java: Essentials for Your Library
Java can be a challenging language to learn, but having the right resources can make all the difference.
The best Java programming books are those that take a variety of approaches to help you learn Java thoroughly.
Head First Java is a great option for beginners, thanks to its gamification features that keep you engaged with learning Java objects.
This book combines puzzles, mysteries, illustrations, and interview-style info with key concepts to help you learn quickly and have fun.
A fresh viewpoint: Learn to Code in Java
Objective-C: Big Nerd Ranch
The Big Nerd Ranch Guide is a great resource for learning Objective-C programming. It's based on the popular Objective-C Bootcamp from Big Nerd Ranch.
This book has an engaging style that makes it easy to learn. It covers C, Objective-C, and provides tips for working with Apple technologies.
Divided into 52 sets of scenarios, tips, and shortcuts, this book is concise and practical. You'll learn how to avoid little-known pitfalls and choose the best option possible.
If you're a beginner, you might want to start with the iOS Programming: The Big Nerd Ranch Guide. It teaches you the foundations of iOS development and the tools you need to create awesome features.
The Pragmatic Programmer: From Journeyman to Master
The Pragmatic Programmer: From Journeyman to Master is a must-read for any software developer looking to take their skills to the next level. This book, written by Andrew Hunt and David Thomas, has been a consistent bestseller since its release in 1999.
The book provides valuable advice for software developers, weaving in short stories and anecdotes to make the material more engaging and memorable. A new edition, The Pragmatic Programmer: your journey to mastery, was released in September 2019 to celebrate the book's 20th anniversary.
This updated edition includes extensive overhauls to the source material, with about a third of the book being entirely new information. The rest of the text has been edited for clarity, making it an even more valuable resource for developers.
Here are some key takeaways from the book:
- Learn core processes that enable you to be a better programmer and produce flexible, efficient code.
- Get tips for honing your career skills, from work ethic to common pitfalls to avoid.
If you're looking to improve your coding skills and become a master programmer, The Pragmatic Programmer is an excellent resource to add to your reading list.
A Brain-Friendly
If you're a visual learner, you'll love "brain-friendly" guides that make learning to code a breeze. These guides are packed with illustrations and examples that help you grasp complex concepts quickly.
One of the best examples is the "Head First Python" guide, which uses a brain-friendly approach to teach you Python concepts and build your own web application.
The "Head First Android Development" guide is another great example of a brain-friendly guide, with plenty of visual illustrations to help you learn and keep you engaged.
Related reading: Learn How to Code Tetris Game Python
The "Head First Kotlin" guide takes a similar approach, teaching you how to think like a great Kotlin developer through a conversational and engaging writing style.
These guides are perfect for those who learn best through visual aids and interactive design, and they're a great way to improve your coding skills and build working apps.
R Cookbook: Proven Recipes for Data Analysis and Graphics
The R Cookbook is a must-have for anyone looking to improve their data analysis skills with R. It contains over 200 practical R "recipes" that you can experiment with.
This cookbook is designed to be a hands-on guide, allowing you to learn by doing. You'll be able to tackle tasks like input and output, work with statistics, and more as you analyze data.
One of the standout features of the R Cookbook is its accessibility. The author, Paul Teetor, has made sure that the book is easy to follow, even for those who are new to R or programming in general.
On a similar theme: Learn to Code in R
SQL Queries for Mere Mortals: A Hands-On Guide
If you're new to SQL, a great place to start is with "SQL Queries for Mere Mortals: A Hands-On Guide to Data Manipulation in SQL". This code book provides a clear, simple tutorial on creating reliable SQL queries for modern databases.
It covers every aspect of query writing, so you can write effective ones of all types. The book is written by Dan Vanderkam and is available on Amazon.
This book is perfect for those who want to learn SQL from scratch, as it breaks down complex concepts into easy-to-understand language. Its comprehensive approach will have you writing SQL queries like a pro in no time.
The book's author, Dan Vanderkam, has clearly done his research and provides a thorough guide to SQL queries. His expertise shines through in the book's clear and concise explanations.
Frequently Asked Questions
Which book is best for programming for beginners?
For programming beginners, "The Programmer's Brain" by Felienne Hermans is a great starting point, offering practical insights into how programmers think and learn. It's a gentle introduction to programming concepts and best practices.
Can I learn coding by books?
Yes, you can learn coding by books, as they often provide well-organized content that helps beginners learn in a progressive and easy-to-follow manner. Check out our list of top coding books for beginners to get started!
What books to read to learn coding?
To learn coding, consider reading "The Self-Taught Programmer" for a comprehensive guide, "The Pragmatic Programmer" for expert-level advice, and "Learning JavaScript Design Patterns" for practical skills. These books cover essential topics for coding professionals, from basics to advanced techniques.
Sources
- https://www.codecademy.com/learn/learn-how-to-code
- https://blog.hubspot.com/website/how-to-start-coding
- https://daily.dev/blog/beginners-guide-how-to-start-learning-coding-from-scratch
- https://devmountain.com/blog/7-highly-recommended-programming-books/
- https://learntocodewith.me/posts/programming-books/
Featured Images: pexels.com