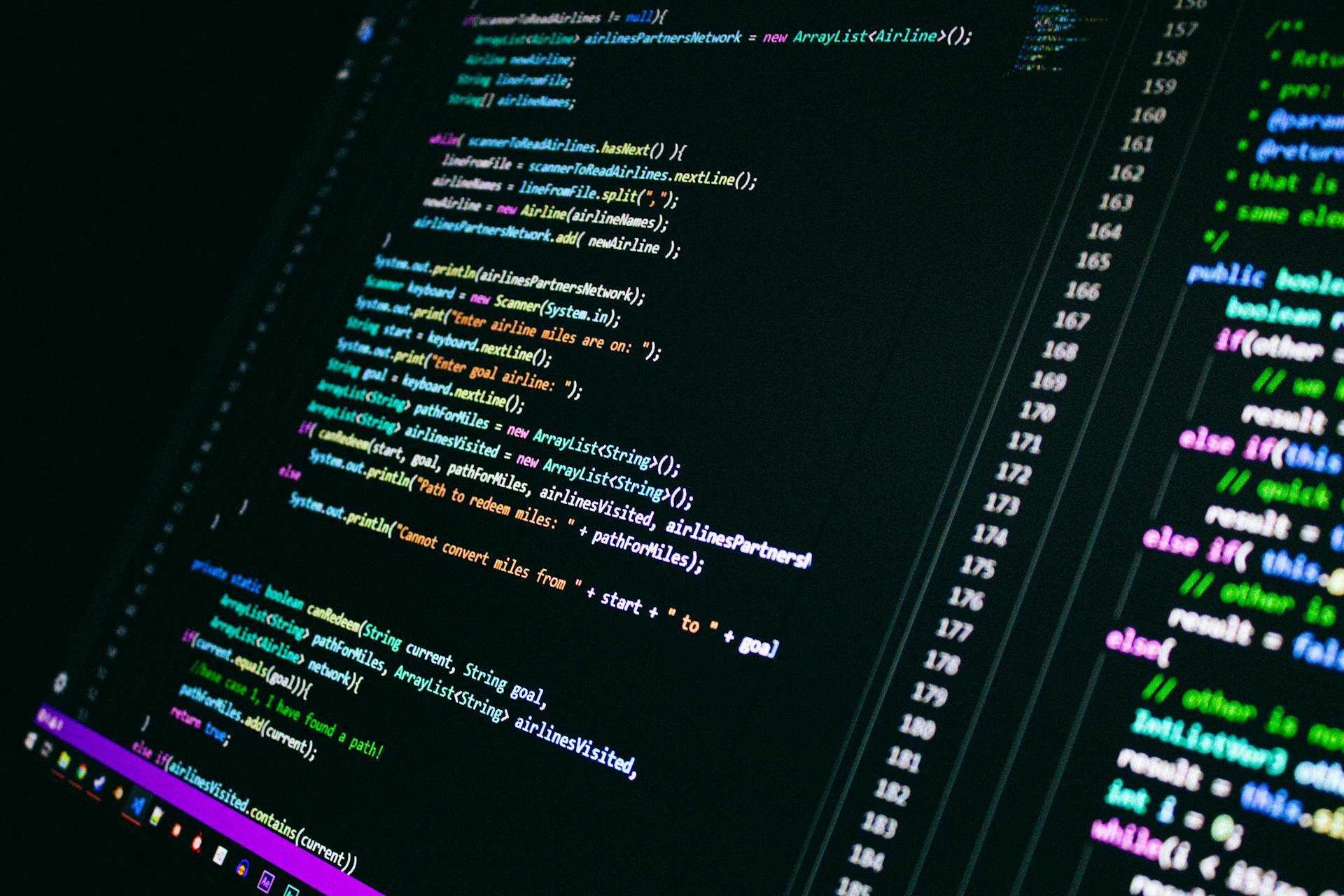
Learning to code in Java can be a daunting task, but with a solid foundation and a clear understanding of the basics, you can start building your skills and confidence. Java is an object-oriented language that's widely used in Android app development, web development, and enterprise software development.
To begin with, you'll need to understand the basic syntax of Java, including variables, data types, operators, and control structures. This will form the building blocks of your Java skills, allowing you to write simple programs and scripts.
As you progress, you'll learn about object-oriented programming (OOP) concepts, such as encapsulation, inheritance, and polymorphism. These concepts will help you organize and structure your code in a more efficient and maintainable way.
With practice and patience, you'll be able to tackle more advanced topics, including multithreading, networking, and database connectivity.
A fresh viewpoint: Books to Help Learn Code in Java
Getting Started
To get started with learning Java, you'll want to understand the basics of object-oriented programming. This includes concepts like objects, classes, interfaces, packages, and inheritance.
Start by defining your own classes, declaring member variables, methods, and constructors. This will help you build a strong foundation in Java programming.
Java is an object-oriented language, which means it's all about organizing code into objects that have properties and behaviors. You'll learn about classes and objects, and how to use them to create robust and maintainable code.
You'll also want to learn about numbers and strings, which are fundamental data types in Java. Understanding how to work with these data types will help you write more efficient and effective code.
Here's a quick rundown of the key concepts you'll need to get started:
By understanding these concepts, you'll be well on your way to becoming proficient in Java programming. Remember to practice regularly and experiment with different coding techniques to reinforce your learning.
Java Fundamentals
Java is a powerful and versatile programming language that has stood the test of time since its inception in the mid-1990s. Developed by Sun Microsystems (now owned by Oracle), Java has become a cornerstone in the world of software development.
To get started with Java, you'll want to learn the basics of programming, including classes, APIs, and deployment. Oracle's Java Tutorials covers these topics, including classes in Java, Java APIs, and deploying applications.
You can also explore other resources, such as Java Beginners Tutorial, which provides a comprehensive guide to learning Java, including topics like Java HashMap, LinkedList, and Set. Another useful resource is Tutorials Point, which offers a wide range of tutorials on Java and other programming languages.
Here are some key concepts to get you started:
- Classes in Java
- Java APIs
- Deploying applications
- Java HashMap
- LinkedList
- Set
Variables
Variables are a fundamental concept in Java programming, used to store and manipulate data within your code. They can hold data of different types, like numbers or text.
A variable is a container that holds a value, as explained in Example 16, "1. Variables". There are two types of variables: primitive variables, which store data directly, and reference variables, which hold a link to an object from a class.
Variables can be declared as local or instance variables. Local variables are declared within a method or block and only work within that same place, while instance variables are inside a class but outside any method, and their values change with each class instance.
Here are some key points about variables in Java:
- Variables can hold data of different types, like numbers or text.
- There are two types of variables: primitive variables and reference variables.
- Variables can be declared as local or instance variables.
In Example 16, "1. Variables", it's mentioned that variables can be used to store data of different types. This is a crucial aspect of programming, as it allows you to work with different types of data in your code.
By understanding how variables work in Java, you'll be able to write more efficient and effective code. So, make sure to practice using variables in your Java programs to get a good grasp of this fundamental concept.
Readers also liked: Is Transfer Learning Different than Deep Learning
Exception Handling
Exception handling is a crucial part of Java programming that helps deal with errors that occur while the program runs.
In Java, you create an object for each error that occurs in a method, which is part of the object-oriented style of this language that helps with spotting and fixing errors so they don't stop the program.
You put code that might have an error in a "try" block. If an error does happen, the program makes an exception object and throws it out of the try block.
Some good habits when using exceptions include careful logging and not hiding thrown exceptions from view by catching them too early or at a too low level.
Here are some key concepts related to exception handling in Java:
- Java Exceptions
- Java Try-catch block
- Java Multiple Catch Block
- Java Nested try
- Java Finally Block
- Java Throw Keyword
- Java Exception Propagation
- Java Throws Keyword
- Java Throw vs Throws
- Final vs Finally vs Finalize
- Exception Handling with Method Overriding
- Java Custom Exceptions
Advanced Programming
As you progress in your Java programming journey, you'll encounter more complex concepts that will help you tackle real-world projects.
Advanced Java programming concepts include file handling, which allows you to read and write data to files, making it easy to store and retrieve information.
File handling is a crucial aspect of Java programming, enabling you to work with files and directories, whether it's reading data from a file, writing data to a file, or deleting a file.
Data structures such as lists, queues, maps, and sets are also essential for advanced Java programming, helping you organize and manipulate data efficiently.
Broaden your view: Learn How to Code Google's Go Golang Programming Language
These data structures can be used to solve complex problems, such as finding duplicates in a list or determining the frequency of each item in a set.
Input/output streams are another vital concept in Java programming, allowing you to read from and write to various sources, such as files, networks, and devices.
The Java reader/writer functionality provides a convenient way to work with text files, making it easy to read and write data in a structured format.
Java Concepts
Java is an object-oriented programming language that's widely used for developing large-scale applications. It's designed to be platform-independent, meaning you can write code once and run it on any device with a Java Virtual Machine (JVM) installed.
In Java, variables are declared with a specific data type, such as int, double, or String, which determines the type of value it can hold. The data type also determines the amount of memory allocated to the variable.
Java uses a concept called encapsulation, where data is hidden within a class and can only be accessed through methods. This helps to protect the data from being modified accidentally or maliciously.
Reader/Writer
In Java, Reader/Writer is an essential concept for handling text data in web applications. It's a higher-level abstraction that provides a character-based approach to Input/Output (I/O) operations.
Java Reader/Writer is designed to handle character-oriented data, which is different from byte-oriented data handled by InputStream and OutputStream. This makes it a valuable tool for working with text in Java.
Reader and Writer are abstract classes that provide a minimal implementation dependency, making them compatible with different platforms. This flexibility is a significant advantage in Java development.
Java Reader/Writer is used to handle reading and writing data, offering a more efficient way to work with text compared to byte-oriented data.
Object
In Java, an Object is the basic building block of a program. It represents a real-world entity or a concept.
An Object is an instance of a Class, which means it has its own set of attributes and methods. For example, a Car Class can have attributes like color, model, and year, and methods like startEngine() and accelerate().
Here's a summary of key concepts related to Objects in Java:
- Object is an instance of a Class.
- Object has its own set of attributes and methods.
In Java, you can create an Object using the new keyword, followed by the Class name. For example: Car myCar = new Car();
Polymorphism
Polymorphism is a fundamental concept in Java programming that allows for more flexibility and reusability of code. It's a powerful feature that enables objects of different classes to be treated as objects of a common superclass.
Method Overloading is a form of polymorphism where multiple methods with the same name can be defined, but with different parameters. This allows for more flexibility in method calls.
Method Overriding is another form of polymorphism where a subclass provides a different implementation of a method that's already defined in its superclass. This allows for more specific behavior in subclasses.
Covariant Return Type is a feature of polymorphism that allows a subclass to return a more specific type than its superclass. This is useful when a subclass wants to return a more specific type of object.
The super keyword is used in polymorphism to access members of a superclass from a subclass. It's like a shortcut to the superclass.
Take a look at this: Feature Learning
Instance Initializer blocks are used in polymorphism to initialize instance variables before the constructor is called. This is useful when you need to perform some initialization before the object is created.
The final keyword can be used in polymorphism to prevent a method from being overridden in a subclass. This ensures that the method is always called with the same behavior.
Runtime Polymorphism occurs when the correct method to call is determined at runtime, rather than at compile time. This is useful when you want to call a method based on the actual object type, rather than the declared type.
Dynamic Binding is a technique used in polymorphism to resolve method calls at runtime. It's like a lookup table that determines which method to call based on the object type.
The instanceof operator is used in polymorphism to check if an object is an instance of a particular class. This is useful when you want to perform different actions based on the object type.
Abstraction
Abstraction is a fundamental concept in Java that helps us write more organized and efficient code.
In Java, abstraction is achieved through the use of abstract classes and interfaces.
Abstraction allows us to hide the implementation details of an object from the user, showing only the necessary information.
For example, when using a bank account, you don't need to know how the bank stores your account information, you only need to know how to deposit and withdraw money.
This is achieved through the use of methods in Java, which encapsulate a set of instructions that can be executed to perform a specific task.
In the example of the BankAccount class, the deposit and withdraw methods hide the implementation details of how the account balance is updated.
Encapsulation
Encapsulation is a fundamental concept in object-oriented programming that helps keep data private and secure.
In Java, encapsulation is achieved by declaring variables as private and providing public methods to access and modify them.
This approach prevents external classes from directly accessing or modifying an object's internal state, which helps maintain data integrity and security.
Encapsulation also promotes code reusability by allowing classes to be designed with a well-defined interface and internal implementation.
By hiding the internal implementation details, encapsulation makes it easier to modify or replace the implementation without affecting other parts of the code.
For example, in the "Classes and Objects" section, we saw how encapsulation can be used to create a simple BankAccount class with private variables for balance and account number.
The BankAccount class provides public methods to deposit and withdraw money, ensuring that the account balance is always updated correctly and securely.
Synchronization
Synchronization is a crucial concept in Java that allows multiple threads to access shared resources safely. It's a mechanism to prevent data inconsistency and ensure that only one thread can access a resource at a time.
Synchronization in Java is achieved using the synchronized keyword, which can be applied to a block of code, a method, or an entire class. The synchronized block is a specific section of code that is locked while it's being executed, preventing other threads from accessing it.
Deadlock in Java occurs when two or more threads are blocked indefinitely, each waiting for the other to release a resource. This can happen when multiple threads are competing for shared resources.
Reentrant lock is a type of lock that can be acquired multiple times by the same thread without causing a deadlock. This is useful in situations where a thread needs to access a resource multiple times.
Here are some common synchronization techniques in Java:
- Synchronized block
- Static synchronization
- Reentrant Monitor
These techniques can be used to prevent data inconsistency and ensure that multiple threads can access shared resources safely. By using synchronization correctly, you can write efficient and thread-safe code that's easy to maintain and debug.
Networking
Networking in Java is a vast and complex topic. It involves understanding how devices on a network communicate with each other.
Networking concepts are the foundation of Java Networking. They include understanding protocols, devices, and the flow of data over a network.
Socket programming is a crucial aspect of Java Networking. It allows you to create a connection between two devices over a network, enabling communication.
The URL class is used to create a URL object, which represents a Uniform Resource Locator. This is a string that identifies a resource on the internet.
The URL class is used to create a URL object, which is then used to connect to a resource on the internet. This is a fundamental concept in Java Networking.
The URLConnection class is used to establish a connection to a resource on the internet. It provides methods for reading and writing data over the connection.
The HttpURLConnection class is a subclass of URLConnection that provides additional methods for making HTTP requests over the connection.
The InetAddress class is used to represent an IP address in Java. This is a string that identifies a device on a network.
Here's a list of some key concepts in Java Networking:
- Socket programming
- URL class
- URLConnection class
- HttpURLConnection
- InetAddress class
Reflection
Reflection is a powerful feature in Java that allows you to inspect and dynamically call classes, methods, and fields at runtime.
The Reflection API is a key part of this feature, providing a way to access and manipulate classes, methods, and fields.
You can use the newInstance() method to create a new instance of a class.
The javap tool is a useful utility that can be used to view the structure of classes and methods.
Creating a custom javap tool can be a fun and educational project.
You can use the appletviewer tool to view and test applets.
Calling private methods using reflection can be a bit tricky, but it's possible with the right approach.
Here are some key methods and tools related to reflection:
- Reflection API
- newInstance() method
- javap tool
- appletviewer
Java RMI, or Remote Method Invocation, is another important concept in Java that allows you to call methods on remote objects.
JDBC
JDBC is a Java API that allows you to connect to a database and execute SQL queries. It's a crucial part of any Java application that interacts with a database.
The JDBC API is made up of several key components, including the JDBC Driver, which is responsible for connecting to the database, and the DriverManager, which manages the connections.
To connect to a database using JDBC, you need to follow several steps, including establishing a connection, creating a statement, and executing a query. The DB Connectivity Steps outlined in the JDBC section provide a clear guide on how to do this.
One of the most common databases used with JDBC is Oracle, and the Connectivity with Oracle section of the article provides a detailed guide on how to connect to an Oracle database using JDBC.
JDBC also provides several ways to interact with a database, including using a Statement, which executes a query, and a ResultSet, which provides the results of the query.
Here's a quick rundown of the key components of JDBC:
Prepared Statements are also a key part of JDBC, and the Prepared Statement section of the article explains how to use them to execute queries with parameters.
Transaction Management is also an important aspect of JDBC, and the Transaction Management section of the article provides a guide on how to manage transactions using JDBC.
Overall, JDBC is a powerful tool for interacting with databases in Java, and understanding its key components and features is essential for any Java developer working with databases.
Best Practices
As you learn to code Java, it's essential to follow best practices that will make your coding journey smoother and more efficient. Experienced developers have an abundance of knowledge and practical experience, and learning from them can benefit you greatly.
Learning from industry best practices, coding conventions, and design patterns early on can set a solid foundation for your coding style and professionalism. Seasoned developers can guide you on common pitfalls and mistakes that learners often make, helping you avoid unnecessary challenges.
Getting feedback on your code from experienced developers is invaluable, as they can provide constructive criticism, point out areas for improvement, and help you write cleaner, more maintainable code.
Related reading: Best Code to Learn
Practise Coding Constantly
Practising coding constantly is essential to improve your skills. Regular practice helps you develop and refine your coding skills over time.
Coding challenges and exercises provide an opportunity to enhance your problem-solving. You become proficient at breaking down complex problems into smaller, manageable steps.
Regular coding practice encourages you to think algorithmically. This helps you design efficient algorithms to solve complex problems.
The more you code, the more confident you become in your abilities. Overcoming coding challenges boosts your confidence to tackle more complex problems and projects.
A unique perspective: Claude Ai Coding
Stay Current
Staying current in the Java programming field is crucial for enhancing your programming skills and staying competitive. You can start by keeping up with the latest news and trends.
Java has come a long way since Java 8, and it's essential to be aware of its evolution. New versions of Java are released regularly, and knowing about the updates will enable you to leverage the latest features and improvements in your projects.
To stay current, you can use the preview features available in the JDK. These features allow you to compile and execute new functionality, giving you a head start on the latest technology.
Staying informed about software frameworks like Spring Framework 6 can expand your capabilities as a developer. This means you can take advantage of new tools and techniques to improve your projects.
Here are some areas to focus on to stay current:
- Java Platform Evolution: Understand the latest changes and updates to the Java platform.
- Preview Features: Learn how to compile and execute the preview features available in the JDK.
- Software Frameworks: Stay up-to-date with the latest versions of software frameworks like Spring Framework 6.
- Industry Trends: Keep informed about the latest news and trends in the Java programming field.
Seek Guidance from Experienced Developers
Connecting with experienced developers is a game-changer for your coding journey. They can guide you on common pitfalls and mistakes that learners often make, helping you avoid unnecessary challenges and adopt better coding practices.
Experienced developers are familiar with industry best practices, coding conventions, and design patterns. Learning these early in your journey can set a solid foundation for your coding style and professionalism.
Networking with other developers opens doors to job opportunities, project collaborations, and mentorship. This is especially true for Java developers who can tap into online coding communities and forums.
Getting feedback on your code from experienced developers is invaluable. They can provide constructive criticism, point out areas for improvement, and help you write cleaner, more maintainable code.
Latest Courses
Learning to code in Java can be a daunting task, but with the right resources, you can master it in no time. There are numerous online platforms that offer Java tutorials and courses.
Codecademy, Udemy, and Coursera are just a few of the many platforms that provide comprehensive Java courses. You can also check out Java Code Geeks, Learn Java, and Oracle Java Tutorials for in-depth learning materials.
If you're looking for a more interactive learning experience, consider using SoloLearn, Skillshare, or Codementor. These platforms offer a range of Java courses and tutorials, from beginner to advanced levels.
Here are some of the top platforms for learning Java:
In addition to these platforms, you can also explore other resources such as Java for Android, Java Fundamentals, and Advanced Java courses.
Frequently Asked Questions
Is Java easy to learn?
Java is relatively easy to learn for those with prior programming experience, but beginners may find its syntax challenging to grasp at first
Can I learn Java coding for free?
Yes, you can learn Java coding for free, with numerous online courses available. Start exploring free resources to begin your Java learning journey today.
Featured Images: pexels.com