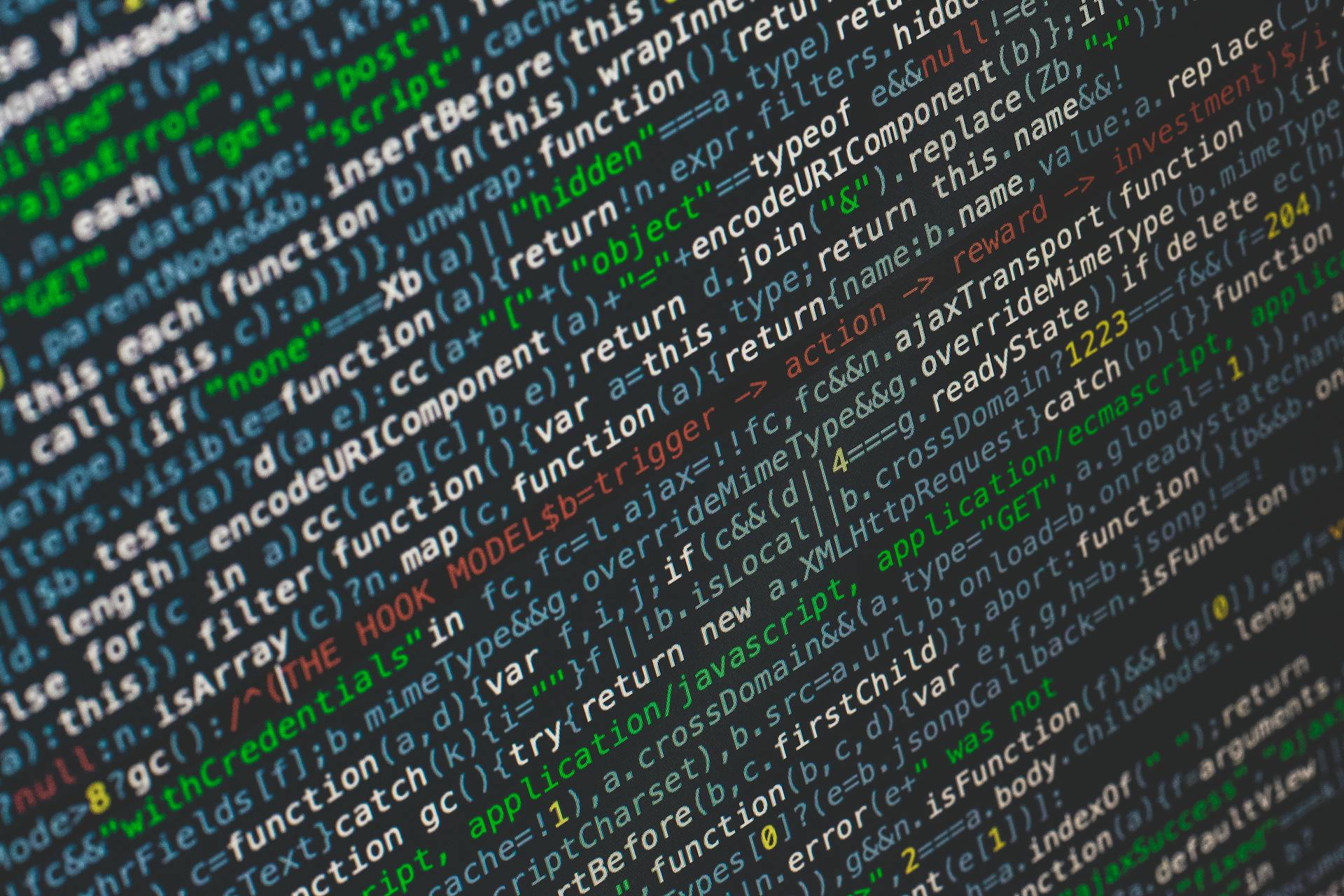
Learning JavaScript can seem daunting, but mastering its fundamentals is key to unlocking a world of possibilities. JavaScript is a high-level, dynamic language that can be used for both front-end and back-end development.
One of the most important things to understand is the difference between var, let, and const. According to the article, var is function-scoped, while let and const are block-scoped. This can greatly impact the way you write and debug your code.
Understanding the basics of data types is crucial for any JavaScript developer. JavaScript has five primitive data types: number, string, boolean, null, and undefined. These data types are the foundation upon which more complex data structures are built.
With practice and patience, you can master the basics of JavaScript and start building complex web applications.
Getting Started
If you're new to coding, it's exciting to start learning JavaScript. This versatile programming language is used on both server-side and client-side to build interactive web pages, applications, and games.
There are many free resources available to learn JavaScript, including YouTube courses. For example, the freeCodeCamp YouTube course has over 7 million views and covers basic JavaScript challenges.
To get started, you can choose from a variety of introductory courses, such as the Traversy Media YouTube course or the Programming with Mosh course. These courses will walk you through the basics of JavaScript, including conditions, loops, functions, objects, arrays, and ES6 features.
Some popular introductory courses include:
Learning JavaScript can be a fun and rewarding experience, and with the right resources, you can get started quickly. So, take the first step and start exploring the world of JavaScript today!
Course Structure
The course structure of a JavaScript learning program can vary, but some popular courses offer a comprehensive approach to learning the language.
The freeCodeCamp YouTube course has over 7 million views on YouTube, indicating a well-structured and engaging learning experience.
In this course, you'll learn about conditions, loops, functions, objects, arrays, and ES6, covering the basics of JavaScript.
Some courses, like the SoloLearn JavaScript Course, offer dozens of challenges, quizzes, and projects to help you practice and retain the material.
You'll have the opportunity to work on projects that apply your knowledge, such as building a JavaScript DOM project.
The Traversy Media YouTube course, on the other hand, focuses on providing a short and introductory course on JavaScript basics.
Here are some specific projects you'll work on in the Traversy Media course:
- JavaScript DOM Crash Course - Part 1
- JavaScript DOM Crash Course - Part 2
- Fetch API Introduction
- AJAX Crash Course (Vanilla JavaScript)
- JavaScript OOP Crash Course (ES5 & ES6)
- JSON Crash Course
Algorithms and Data Structures
Learning algorithms and data structures is a crucial part of becoming proficient in JavaScript. In the freeCodeCamp course, you'll learn about basic data structures, which will give you a solid foundation to build upon.
You'll also learn about Object Oriented Programming and functional programming, which are essential skills for any JavaScript developer. Hundreds of challenges will help you practice and reinforce your understanding of these concepts.
By the end of the course, you'll be able to receive a free certification to share on social media or add to your professional profile.
Broaden your view: Learn How to Code Google's Go Golang Programming Language
The Fundamentals
To code effectively with JavaScript, you need to learn the fundamental skills, starting with basic syntax, which is demonstrated with a "Hello, World!" program.
Variables are used to hold data values and are foundational for everything else, so it's essential to know how to declare and initialize variables using the keywords let, const, and var.
Data types in JavaScript include the primitive types string, number, boolean, null, undefined, and object, and being able to cast them to a type explicitly can save you a world of trouble.
You should know how to create and manipulate arrays, which are used to store data in a way that allows you to efficiently access and modify it.
The control flow in JavaScript determines the order in which statements are executed, and you have switch statements, for and while loops, if-else statements, and others available to you.
- Basic syntax: "Hello, World!" program
- Variables: let, const, and var
- Data types: string, number, boolean, null, undefined, and object
- Data structures: arrays
- Control flow: switch statements, for and while loops, if-else statements
Algorithms and Data Structures
Learning algorithms and data structures is a crucial part of programming, and there are many resources available to help you get started.
You can learn JavaScript by doing hundreds of challenges in a freeCodeCamp course, which covers basic JavaScript, ES6, regular expressions, basic data structures, Object Oriented Programming, and functional programming.
This interactive learning environment allows you to learn by doing, making it a more engaging and effective way to learn.
In this course, you'll have the opportunity to receive a free certification to share on Twitter or put on your LinkedIn profile, which can be a great way to showcase your skills to potential employers.
Loops
Loops are a fundamental concept in programming that allow you to execute a set of instructions repeatedly. This repetition is crucial for solving complex problems and making your code more efficient.
In JavaScript, loops are used to control program flow based on conditions, and there are several types of loops to choose from. You can use a for loop to execute a block of code repeatedly, a while loop to execute code as long as a condition is true, or a do...while loop to execute code at least once.
Loops can be used to iterate over arrays, objects, or strings, making them incredibly useful for tasks such as data processing, filtering, and transformation. For example, you can use a for...in loop to iterate over an object's properties or a for...of loop to iterate over an array's elements.
Here are some common types of loops in JavaScript:
- for Loop: executes a block of code repeatedly using a counter
- do...while Loop: executes a block of code at least once and continues as long as a condition is true
- while Loop: executes a block of code as long as a condition is true
- for...in Loop: iterates over an object's properties
- for...of Loop: iterates over an array's elements
Loops are a powerful tool for making your code more efficient and scalable. By using the right type of loop for the job, you can write more concise and readable code that solves complex problems with ease.
Regular Expression
Regular Expression is a powerful tool in programming that allows you to match patterns in strings. Regular expressions are patterns used to match character combinations in strings.
JS Regular expressions, also known as regex or regexp, are a crucial part of JavaScript programming. They can be used to validate user input, parse text, and even replace text within a string.
A Regular Expression is a sequence of characters that combine to form a search pattern. This pattern can be used to match specific characters, numbers, or even a range of characters.
JS Regular expressions have various components, including patterns, flags, metacharacters, and quantifiers. These components help you create complex patterns to match specific text.
Here are some common JS Regular expressions:
- JS RegExp [abc] Expression: Matches any single character that is 'a', 'b', or 'c'
- JS RegExp S Metacharacter: Matches the end of a string or a line in a multi-line string
- JS RegExp m Modifier: Multi-line modifier, which allows the ^ and $ to match the start and end of each line
- JS RegExp ?! Quantifier: Matches the preceding element zero or one time
- JS RegExp {X,Y} Quantifier: Matches the preceding element between X and Y times
- JS RegExp test() Method: Tests whether a string matches a regex pattern
- JS RegExp [^0-9] Expression: Matches any single character that is not a digit
Promises
Promises are a fundamental concept in modern programming, and JavaScript Promises are no exception. They provide a cleaner and more intuitive way to work with asynchronous code compared to traditional callback-based approaches.
JS Promises are particularly useful when dealing with multiple asynchronous operations. You can chain them together to create a sequence of tasks that run one after the other.
JS Errors can be tricky to handle, but with JS Promises, you can use the "throw and try to catch" approach to catch and handle errors in a more elegant way.
In JavaScript, you can even use class compositions to create complex data structures and algorithms. This can be particularly useful when working with large datasets or complex logic.
Here are some key concepts related to JS Promises:
- JS Promise: A promise is a result of an asynchronous operation that may not have completed yet.
- JS Promise Chaining: Chaining promises allows you to create a sequence of tasks that run one after the other.
- JS Errors Throw and Try to Catch: This approach allows you to catch and handle errors in a more elegant way.
- JS Class compositions in JavaScript: Class compositions can be used to create complex data structures and algorithms.
Generators
Generators are a type of function that can pause and resume their execution to produce a sequence of values lazily.
They allow for memory-efficient processing of large datasets by only generating values as needed.
Generators are defined using the function keyword, just like regular functions, but they use the yield keyword to produce values.
This is in contrast to regular functions, which use return to produce a single value and then terminate.
Generators can be used to implement infinite sequences, such as the Fibonacci sequence.
Here's an example of how you might use a generator to produce the Fibonacci sequence:
- JS Function Generator
Object-Oriented Programming
Object-Oriented Programming (OOP) is a concept that enables the structure of code by modeling real-world entities as objects with properties and behaviors.
OOP in JavaScript is about encapsulating data and functions within objects, making it easier to organize and reuse code. This is achieved through classes, which are templates for creating objects. Classes introduce new features useful for object-oriented programming.
Here are some key concepts in OOP:
- Encapsulation: Data is protected from accidental modifications from an outside function.
- Inheritance: Objects can inherit properties and methods from other objects, enabling code reuse.
- Polymorphism: Objects can take on multiple forms, depending on the context.
- Abstraction: Objects can hide their internal implementation details, exposing only the necessary information.
By mastering these concepts, you'll be able to write more efficient, scalable, and maintainable code in JavaScript.
Intermediate Concepts
Intermediate Concepts in Object-Oriented Programming are crucial to take your skills to the next level.
You'll want to make sure you understand the following advanced concepts: Strict mode, which has different semantics to regular JavaScript code and will throw errors that would otherwise be silent.
The this keyword is an important concept that you'll need to wrap your head around in JavaScript. It refers to an object, but it will refer to different objects depending on how it's used.
Asynchronous programming enables programs to start tasks while still remaining responsive to other events, rather than having to wait for tasks to be finished.
A different take: Is Transfer Learning Different than Deep Learning
Here are some key intermediate concepts to focus on:
- Strict mode: Enforces a more restrictive syntax and throws errors for silent errors.
- The this keyword: Refers to different objects depending on the context.
- Asynchronous programming: Allows programs to run tasks in parallel and remain responsive.
- Classes: A template for creating objects with encapsulated data and code.
- Working with APIs: Interacting with external services to add functionality to your projects.
- Debugging: Troubleshooting code to identify and fix errors.
- Version control: Using Git to manage changes and collaborate with others.
Understanding these concepts will help you write more robust, efficient, and scalable code, and take your Object-Oriented Programming skills to the next level.
Best Practices
In Object-Oriented Programming, it's essential to follow best practices to write clean and maintainable code.
JavaScript Best Practices suggest learning about the latest best practices when coding with JavaScript, which is a fundamental language in OOP.
To write object-oriented code, it's crucial to keep your functions and variables organized and easy to understand.
Learning about JavaScript Best Practices can help you improve your coding skills and write better OOP code.
Expand your knowledge: What Is the Best Way to Learn to Code
OOPs
Object-Oriented Programming (OOP) is a concept that enables the structure of code by modeling real-world entities as objects with properties and behaviors. This approach helps create organized and maintainable code.
Classes are a fundamental concept in OOP, and in JavaScript, you can create classes using the class construct. This introduces new features that are useful for object-oriented programming. You can also use class expressions, object constructors, and static methods to create classes.
Take a look at this: Unity Learn Create with Code
Here's a breakdown of some key concepts in OOP:
Inheritance allows objects to inherit properties and methods from other objects, enabling code reuse and creating hierarchical relationships between objects. This is achieved through the prototype chain in JavaScript.
JS classes are used to create objects, and they encapsulate data with code to work on that data. This is a key concept in OOP, and it's essential to understand how classes work in JavaScript.
Libraries and Frameworks
Libraries and Frameworks play a vital role in modern web development, offering built-in functions and methods that enhance web pages, making them more dynamic and interactive.
They handle repetitive tasks, allowing developers to focus on core functionality, which is a huge time-saver. By leveraging these functions, developers can create fast and reliable applications with a solid project structure and data flow.
JavaScript libraries provide pre-built solutions for common tasks, allowing developers to save valuable time by not having to write code from scratch. Popular libraries include ReactJS, jQuery, p5.js, D3.js, Collect.js, Underscore.js, Lodash, and Tensorflow.js.
A well-structured library can make a huge difference in the development process, allowing developers to focus on what matters most – building a great application.
Implementing Closures
Implementing Closures is a fundamental concept in Object-Oriented Programming. A closure is a feature in JavaScript where an inner function has access to the variables of the outer function.
Closures help programmers define the scope of functions and what isn't. In JavaScript, closures are particularly useful for encapsulating data and behavior.
By using closures, you can create private variables and functions that are not accessible from outside the outer function. This is achieved by the inner function having access to the variables of the outer function.
In a closure, the inner function can use the variables of the outer function, even after the outer function has returned. This allows for a more flexible and efficient way of organizing code.
Closures are essential for creating objects in JavaScript, as they enable the encapsulation of data and behavior. This is particularly useful for creating objects that need to maintain state over time.
In JavaScript, closures are implemented using inner functions that have access to the variables of the outer function. This allows for the creation of private variables and functions that are not accessible from outside the outer function.
Typeof Overview
The typeof operator in JavaScript is a type-checking tool that returns the data type of a variable, helping you avoid errors before execution.
It's not required to verify variable types when declaring them in JavaScript, but typeof makes it easy to check the data type beforehand.
Typeof can be used with various operands, including variables, literals, and expressions, making it a versatile tool in your programming toolkit.
The typeof operator returns a string indicating the data type of the operand, which can be used to make informed decisions in your code.
In JavaScript, you can use typeof to check the data type of a variable, ensuring you're working with the correct type to avoid errors and bugs.
Typeof is a fundamental operator in JavaScript that helps you understand the data type of a variable, which is essential for writing efficient and effective code.
By using typeof, you can write more robust and reliable code that's less prone to errors and easier to maintain.
Frequently Asked Questions
How can I teach myself JavaScript?
To teach yourself JavaScript, start by enrolling in a coding bootcamp, watching tutorials, reading books, and practicing with projects, while also staying connected with coding communities and listening to industry podcasts. This comprehensive approach will help you gain hands-on experience and stay up-to-date with the latest developments in JavaScript.
Is JavaScript easy to learn?
JavaScript is a beginner-friendly language, making it easy to learn and versatile for various development tasks. Its simplicity and wide range of applications make it a great starting point for developers.
Is 1 year enough to learn JavaScript?
While 1 year is a good start, mastering JavaScript basics can take around 3 months, but continuous learning is essential for long-term proficiency. With dedication, you can make significant progress in a year, but be prepared to keep learning beyond that.
Is 2 months enough to learn JavaScript?
While 2 months can get you started with JavaScript basics, achieving advanced proficiency typically requires 6-9 months of consistent practice and learning. If you're looking to master JavaScript, it's best to plan for a longer learning period.
Sources
- https://www.freecodecamp.org/news/learn-javascript-free-js-courses-for-beginners/
- https://www.codingdojo.com/blog/how-to-learn-javascript
- https://blog.jetbrains.com/webstorm/2024/07/how-to-learn-javascript/
- https://www.geeksforgeeks.org/javascript/
- https://www.simplilearn.com/tutorials/javascript-tutorial
Featured Images: pexels.com