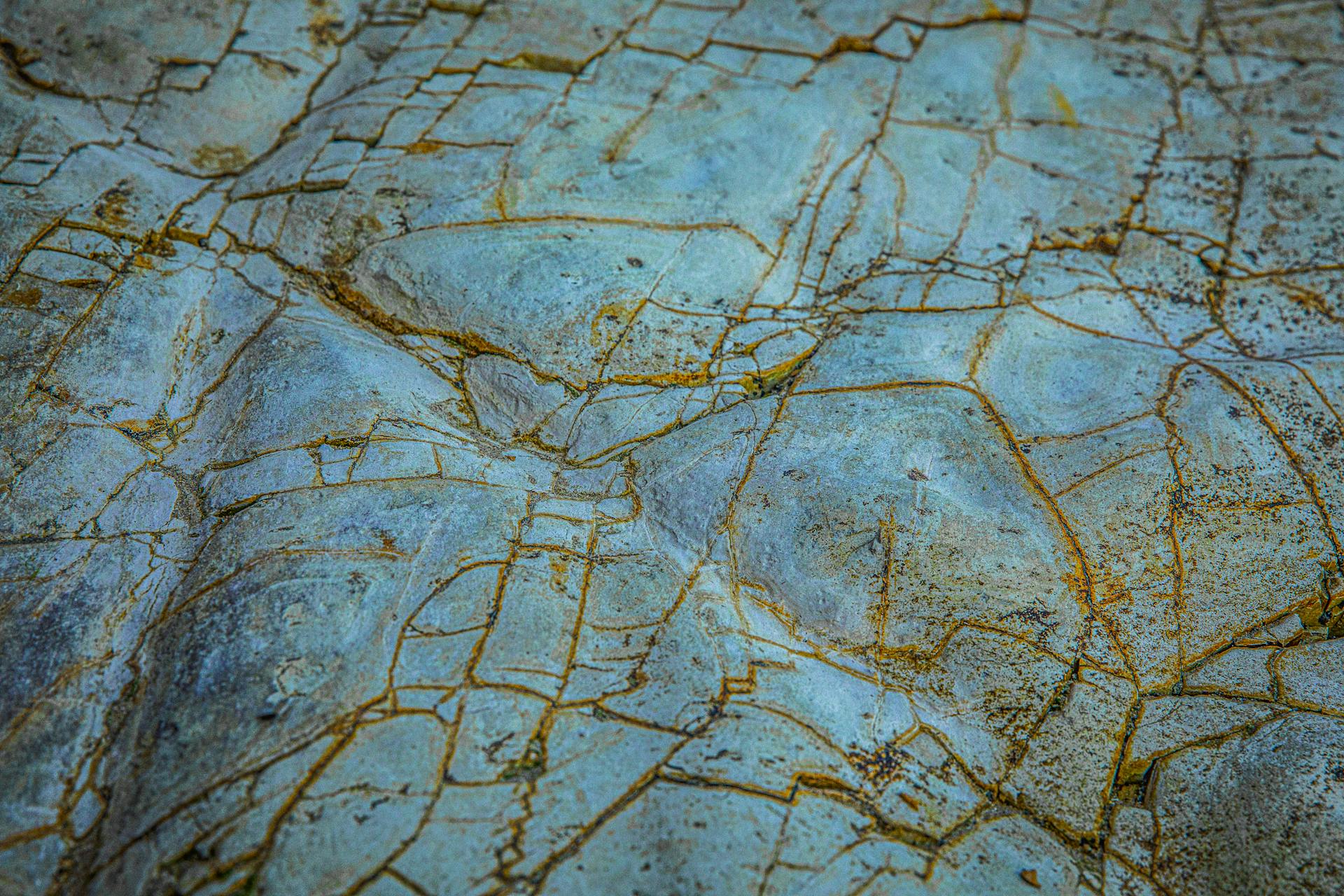
Rust is a modern, systems programming language that's gaining popularity due to its memory safety features and performance.
Rust's ownership system is a key concept to grasp, where every value in Rust has an owner and an optional borrow checker ensures memory safety.
Getting started with Rust is a breeze, thanks to the official Rust installation guide, which provides step-by-step instructions for setting up the language on your computer.
Rust's syntax is designed to be concise and easy to read, with a focus on expressing the programmer's intent clearly.
A unique perspective: What Is the Hardest Code Language to Learn
Getting Started
To get started with Rust, you'll want to set up and use it on your computer for larger development projects. This will give you a solid foundation to build upon.
The Beginner's Guide to Rust provides tutorials that will prepare you for the next step in your career. It covers topics such as Rust Print Output, Variables and Mutability, and Data Types.
Rust Print Output is a fundamental concept that allows you to print text to the screen. You can use the `println!` macro to print output.
Variables and Mutability in Rust are crucial concepts to understand. You can declare variables with the `let` keyword and use the `mut` keyword to make them mutable.
The Rust Programming: The Complete Developer’s Guide by ZTM covers computer programming fundamentals and Rust’s programming language concepts, including traits, slices, memory, generics, and mutability. This comprehensive guide will take you from absolute beginner to learning the fundamentals of programming.
Getting Started with Rust requires setting up the Rust ecosystem, which includes the Cargo Package Manager. Cargo is a package manager that helps you manage dependencies and build your projects.
Beginner Rust Programming by Ricc Messier covers the basic Rust concepts and real-world practical examples. This book is ideal for beginners with some basic C/C++ knowledge and those starting with programming.
For more insights, see: Learn How to Code Google's Go Golang Programming Language
Variables and Data Types
In Rust, you can declare variables using the let, const, or static keywords. Variables are immutable by default, but you can make a variable mutable by using the mut keyword.
Rust convention relies on the following casing conventions for variables, which are declared in snake_case. This helps keep your code organized and easy to read.
Here's a quick rundown of the casing conventions in Rust:
Since Rust is statically typed, you'll need to explicitly type variables – unless the variable is declared with let and the type can be inferred. This ensures that your code is safe and efficient.
Variables
Variables in Rust are declared using the let, const, or static keywords. By default, all variables are immutable, but you can make a variable mutable by using the mut keyword.
You can explicitly type variables in Rust, unless the variable is declared with let and the type can be inferred. This is because Rust is statically typed.
Additional reading: Can I Generate Code Using Generative Ai Models
Variables should follow Rust's casing conventions, which rely on snake_case for variables, functions, and files. Constants and statics, on the other hand, use SCREAMING_SNAKE_CASE.
Here are the casing conventions for different types of objects in Rust:
Rust also uses the underscore before a variable name to indicate that the variable is unused.
The Character Type
A char in Rust is a USV (Unicode Scalar Value), which is represented in Unicode with values like U+221E – the Unicode for '∞'.
In Rust, you can think of a collection or array of chars as a string.
A char is a single character, like 'a' or '∞', and it's used to represent a single Unicode code point.
Rust represents chars in Unicode, which is a standard for encoding, representing, and processing text.
Chars are often used to represent single characters in a string.
Number Types
Number Types in Rust are quite straightforward. There are many types of numbers to choose from, including unsigned integers and signed integers.
Unsigned integers can only represent positive whole numbers, which means they're perfect for counting up, but not for counting down or representing negative numbers.
For example, the u8 type can represent numbers from 0 to 255.
Signed integers, on the other hand, can represent both positive and negative whole numbers. This makes them super useful for a wide range of applications.
Here's a quick rundown of the different types of numbers in Rust:
- Unsigned Integers: u8, u16, u32, u64, u128
- Signed Integers: i8, i16, i32, i64, i128
- Floating Point Numbers: f32, f64
Floating point numbers, like f32 and f64, are used for decimal numbers and are a must-have for any serious math or science project.
Strings and Slices
Strings in Rust are a bit tricky, especially for beginners.
A common point of confusion is the difference between the String struct and the str type.
Strings in Rust can be stored in two ways: stack stored or heap allocated.
my_str, a reference to a string literal, is stack stored.
my_string, an instance of the String struct, is heap allocated.
This means its size can be unknown at compile time.
A string literal is also known as a string slice.
This is because a &str refers to part of a string.
Arrays and strings are similar in that they both have a fixed or unknown size.
The [T; n] notation is used to create an array of n elements of type T.
Language
Rust is a powerful language that's perfect for creating scalable and reliable software. It's a popular choice among developers, with over 50,000 copies of "The Rust Programming Language" book sold.
The Rust language has a unique syntax, with a [dependencies] heading that denotes the crates your project depends on, which are like external libraries. The language also has a built-in macro called println! that prints to the console.
One of the key features of Rust is its ability to handle memory safety effectively, which is covered in the Rust Programming Language course by Dmitri Nesteruk. This course is highly-rated on Udemy and covers 8.5 hours of on-demand video.
The Rust language starts with the basics, where you learn how to work with data types, functions, and variables. It's a great language to learn for anyone looking to create reliable software.
Parse Strings
Parsing strings into numbers can be a bit tricky, but it's a crucial step in working with variables of different data types. You can use the parse method of the String struct to achieve this.
To use the parse method, you need to specify the type annotation, and it will return a Result containing the parsed value. The type annotation is used with the turbofish syntax to indicate the type you're trying to parse the string into.
Converting an operator into a char is also necessary for some operations. You can do this by using the chars method on the String struct, which returns an iterator over the characters in the string. The first character can then be unwrapped for use.
Headings
Variables and Data Types are fundamental concepts in programming, and understanding them will help you write more efficient code. The Rust programming language is known for its focus on memory safety and performance.
Rust has a unique approach to memory management, which is essential for writing powerful apps and libraries. Traits in Rust are used to define a set of methods that can be implemented by different types, making it easier to write ergonomic and idiomatic code.
Asynchronous programming is a key concept in modern software development, and Rust provides a robust set of tools for handling concurrency. Declarative and procedural macros are also an essential part of Rust's ecosystem, allowing developers to write more concise and expressive code.
The Rust programming language is designed to be used with larger code bases, making it an ideal choice for complex projects. With Rust, you can write reliable and efficient code that scales with your needs.
Featured Images: pexels.com