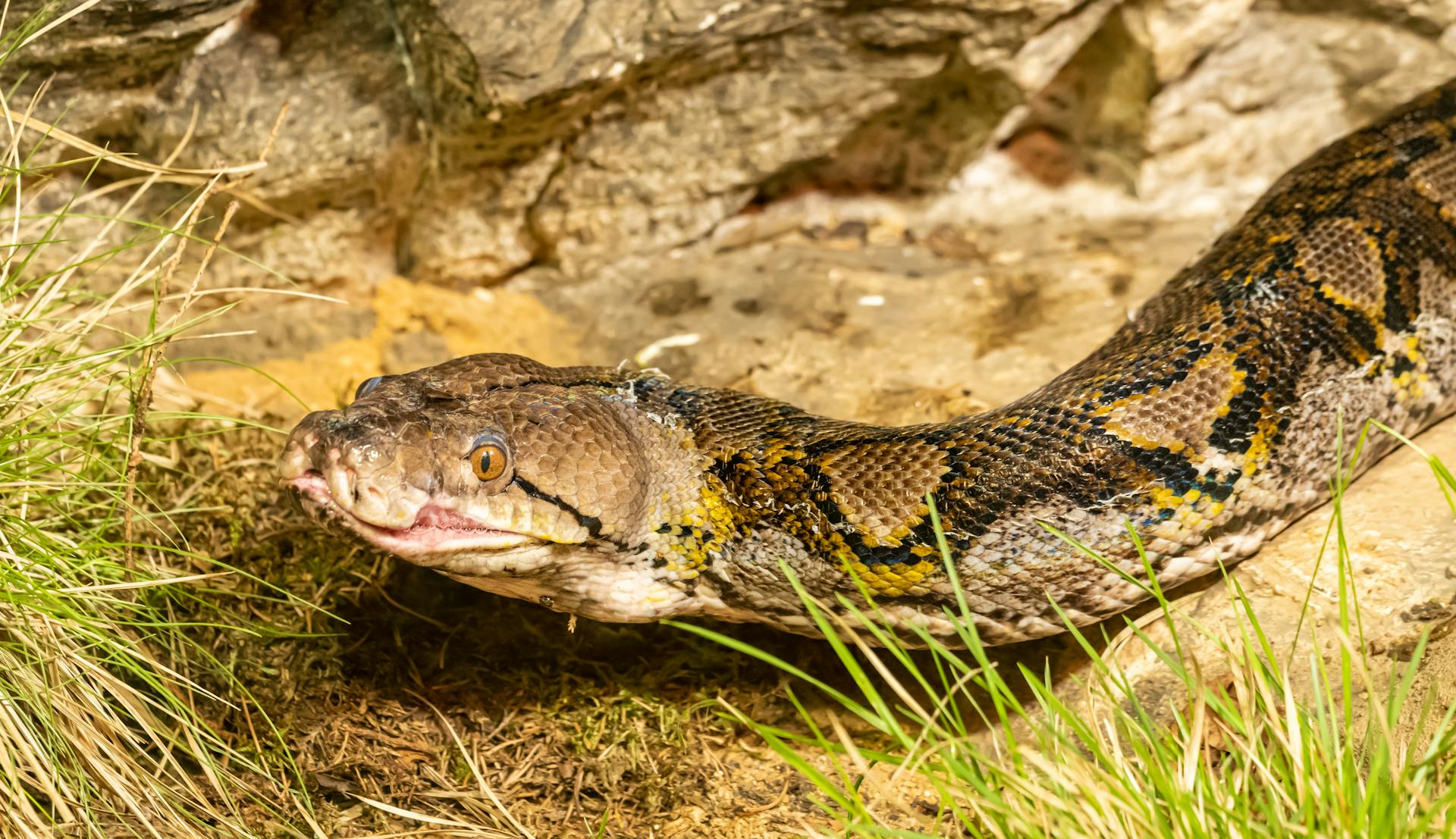
Feature engineering is a critical step in the machine learning pipeline, and Python is an ideal language for implementing it. Python's simplicity and flexibility make it a popular choice among data scientists.
In this comprehensive guide, we'll explore the essential concepts and techniques for feature engineering in Python. We'll start with the basics of data preprocessing, including handling missing values and encoding categorical variables.
Data preprocessing is a crucial step in feature engineering, and Python's libraries such as Pandas and NumPy make it easy to handle missing values. For example, you can use the Pandas library to replace missing values with the mean or median of the respective column.
Feature scaling is another important aspect of feature engineering, and Python's libraries such as Scikit-learn provide efficient algorithms for scaling and normalizing data.
You might enjoy: Hands-on Data Preprocessing in Python
What Is Feature Engineering?
Feature engineering is a machine learning method that uses data to create new variables not included in the training set.
It can generate new features for both supervised and unsupervised learning, making data transformations easier and faster while improving the accuracy of the model.
Feature engineering encapsulates various data engineering techniques such as selecting relevant features, handling missing data, encoding the data, and normalizing it.
This process is crucial in determining the outcome of a model, and it's essential to engineer the features of the input data effectively to ensure the chosen algorithm can perform to its optimum capability.
Feature engineering is the process of using domain knowledge to extract features from raw data that make machine learning algorithms work more efficiently.
It's a critical step in the machine learning pipeline and can significantly impact the performance of models.
To perform feature engineering, a data scientist combines domain knowledge with math and programming skills to transform or come up with new features that will help a machine learning model perform better.
Feature engineering is a practical area of machine learning and is one of the most important aspects of it.
For more insights, see: Scaling Monosemanticity: Extracting Interpretable Features from Claude 3 Sonnet
Benefits of
Feature engineering is a crucial step in machine learning workflows, and it's essential to understand its benefits. Effective feature engineering can lead to better model accuracy, interpretability, and generalization to unseen data.
One of the significant benefits of feature engineering is that it allows for easier algorithms that fit the data. This is because feature engineering transforms raw data into meaningful features that represent the underlying problem to the predictive models.
Feature engineering also enables algorithms to detect patterns in the data more easily. This is because the features created through feature engineering are more relevant and informative, making it easier for algorithms to identify relationships and trends.
By transforming raw data into meaningful features, feature engineering provides greater flexibility of the features. This means that the features can be used in a variety of ways, such as for different machine learning models or for different applications.
Here are some benefits of feature engineering at a glance:
- Higher efficiency of the model
- Easier algorithms that fit the data
- Easier for algorithms to detect patterns in the data
- Greater flexibility of the features
Data Preparation
Data Preparation is a crucial step in feature engineering, and it involves several techniques to handle missing values, encode categorical variables, and transform numerical data. Imputing missing values can be done using mean, median, or mode, but it's essential to choose the right strategy depending on the data distribution.
To handle missing values, you can use SimpleImputer from sklearn.impute, specifying the strategy as a parameter. For example, you can impute missing values with the mean, median, or mode of the corresponding feature columns.
Encoding categorical variables is another important step in data preparation. There are several encoding strategies, including one-hot encoding, label encoding, and binary encoding. One-hot encoding is particularly useful when dealing with categorical variables, as it creates separate columns for each category without creating an ordinal relationship among them.
Here are some common strategies for encoding categorical variables:
In addition to encoding categorical variables, data preparation also involves handling numerical data. Log transformation is a useful technique for centering skewed data, while using domain knowledge can help create new features that improve model performance. For example, you can create a new feature called "Interest elapsed" by calculating the difference between the total due and the loan amount.
Additional reading: Android 12 New Features
Deleting the Columns
Sometimes it's okay to let go of certain features in our dataset if they're not contributing much to the predicted output.
Columns with multiple empty entries or null values can be a problem. If a column has a very high number of null values, it's often best to delete it altogether.
We can fix a threshold value, like 70% or 80%, and if the number of null values exceeds that, we should delete the column.
For example, if a column has 14 null values out of 20 entries, that's not less than our desired threshold, so we delete it.
Deleting columns can reduce dimensionality, which in turn reduces computation complexity.
Here are some common reasons to delete columns:
- High number of null values
- Columns not contributing much to the predicted output
This method is preferred when the dataset is large and deleting a few columns won't affect it much, or when the column to be deleted is a relatively less important feature.
Impute for Variable
Imputing missing values is a crucial step in data preparation. It involves filling in the missing values with some values computed from the corresponding feature columns. There are several strategies for imputing missing values, depending on the type of variable.
For continuous variables, we can use mean, median, or mode to impute missing values. We can use the SimpleImputer from sklearn.impute to specify the imputation strategy and the columns to apply it to.
Imputing with mean is a common strategy, where we replace missing values with the mean value of the corresponding column. Imputing with median is similar, but we use the median value instead. Imputing with mode is another option, where we replace missing values with the most frequent value in the column.
Here are the different imputation strategies for continuous variables:
For categorical variables, we have three main options: dropping the rows containing missing categorical values, assigning a new category to the missing categorical values, or imputing the categorical variable with the most frequent value.
Dropping the rows containing missing categorical values is a simple approach, but it can lead to loss of information. Assigning a new category to the missing categorical values is another option, where we replace the missing values with a new category, such as 'U' for Unknown. Imputing the categorical variable with the most frequent value is also an option, where we replace the missing values with the most frequent value in the column.
You might enjoy: Unsupervised Learning Clustering Algorithms
Here are the different imputation strategies for categorical variables:
In addition to these strategies, we can also use machine learning models to learn the most appropriate fill values. This approach can be more complex, but it can also provide more accurate results.
Handling Outliers
Handling outliers is a crucial step in data preparation, as these values can significantly affect model performance. Outliers can be either mistakes or valuable edge-case information, and it's essential to determine whether to remove or keep them.
To detect outliers, we can use the Inter-quartile range (IQR), which indicates where 50 percent of the data is located. We calculate the IQR by finding the median, then the median of the lower end (Q1) and the median of the higher end (Q3) of the data.
Data between Q1 and Q3 is the IQR, and outliers are defined as samples that fall below Q1 – 1.5(IQR) or above Q3 + 1.5(IQR). We can visualize this using a boxplot, which includes important points like max value, min value, median, and two IQR points (Q1, Q3).
Another way to detect outliers is by using standard deviation, which we can multiply by a factor (usually between 2 and 4) to determine the threshold for outliers. For example, we can use a factor of 2 or 3 to detect outliers.
We can also use percentiles to detect outliers, assuming a certain percentage of the value from the top or bottom as an outlier. The value for the percentiles we use as outliers depends on the distribution of the data.
A unique perspective: Generative Ai with Python and Tensorflow 2 Pdf
Iterative Process
The iterative process of feature engineering is a crucial step in data preparation. It involves continuous refinement of features and models to achieve better results.
Feature engineering is an iterative process that involves continuous refinement. This means that we'll be going back and forth between different steps to improve our features and models.
To illustrate this point, let's take a look at the feature engineering process outlined in the Overview of the Feature Engineering Process. Here are the steps we'll be iterating over:
- Understanding the Data
- Data Preprocessing
- Feature Extraction
- Feature Construction
- Feature Selection
- Model Training and Evaluation
- Iteration
As we work through each step, we'll be refining our features and models to achieve better results. This might involve going back to earlier steps to make adjustments or trying new approaches to improve our features.
In fact, iterative refinement is a key part of the feature engineering process. By continuously refining our features and models, we can improve their accuracy and effectiveness.
Data Transformation
Data transformation is a crucial step in feature engineering, as it helps normalize data and makes it more suitable for analysis. Techniques include log transformation, square root transformation, and box-cox transformation.
These transformations can help reduce skewness in the data and make it more amenable to modeling. For example, log transformation can bring many benefits, including reducing the impact of outliers and making the distribution of the data more normal.
Some common techniques used in data transformation include log transformation, square root transformation, and box-cox transformation. Here are a few examples:
- Log transformation: This involves applying the log function to the current values.
- Square root transformation: This involves taking the square root of the current values.
- Box-Cox transformation: This is a family of power transformations that can be used to stabilize the variance of a distribution.
Imputation for Continuous Data
Imputation for Continuous Data is a crucial step in handling missing values in your dataset. It's a process of filling up the missing values with some values computed from the corresponding feature columns.
You can use a number of strategies for imputing the values of Continuous variables, such as imputing with Mean, Median or Mode. One of the most commonly used imputation methods is to substitute the missing values with the mean value of the feature.
Additional reading: Mlops Continuous Delivery and Automation Pipelines in Machine Learning
To impute with Mean, you'll need to import SimpleImputer from sklearn.impute and pass your strategy as the parameter. This will replace the missing values with the mean values of their corresponding columns.
Alternatively, you can impute with Median by changing the parameter to 'median'. This will replace the missing values with the median values of their corresponding columns.
Another strategy is to impute with Mode, which involves substituting the missing values with the most frequent value in the column. This can be done by passing "most_frequent" as your strategy parameter.
In some cases, you may need to detect missing data before imputing it. You can use Pandas to detect missing values in your dataset. This will help you identify the instances and features with missing values.
For example, you can use Pandas to see which features have missing values, like this: `df.isnull().sum()`. This will give you a count of the missing values in each feature.
Once you've detected the missing values, you can decide whether to drop the samples with missing values or to impute them. If you choose to impute, you can use the mean value of the feature or the most frequent value, depending on the type of feature.
You might enjoy: How Will Ai Affect Software Engineers
Transformation
Data transformation is a crucial step in preparing data for analysis. It helps normalize data and make it more suitable for analysis.
There are several techniques used for data transformation, including log transformation, square root transformation, and box-Cox transformation. These techniques can help make data more normal and reduce the impact of outliers.
Quantile transformation is another technique used to transform data into a uniform or normal distribution. This is especially useful for machine learning algorithms that require data to be in a specific distribution.
Log transformation is a simple yet effective technique that applies the log function to data. It's essential to note that data must be positive before applying log transformation.
Scaling and normalization are also important techniques used in data transformation. They adjust the range of data features, making it easier for machine learning algorithms to work with.
Some common scaling and normalization techniques include Min-Max Scaling, Standardization (Z-score normalization), and Robust Scaler. These techniques can help preserve the distribution of data while scaling it to a common range.
Here are some common data transformation techniques:
- Log transformation
- Square root transformation
- Box-Cox transformation
- Quantile transformation
- Min-Max Scaling
- Standardization (Z-score normalization)
- Robust Scaler
These techniques can help improve the quality of data and make it more suitable for analysis. By applying the right data transformation techniques, you can unlock insights and make better decisions.
On a similar theme: Applied Machine Learning Explainability Techniques
Aggregation
Aggregation is a powerful technique in data transformation that helps create new features from existing data. It's especially useful for time series or grouped data.
Mean, median, and standard deviation of grouped data are all examples of aggregation features that can be created.
Rolling window calculations can also be used to aggregate data, allowing you to analyze trends and patterns over time.
By aggregating data, you can create new features that are more meaningful and useful for analysis.
Here are some common aggregation techniques:
- Mean
- Median
- Standard Deviation
- Rolling Window Calculations
Feature grouping is another important aspect of aggregation, where multiple rows are combined into a single row using aggregation functions. This is especially useful when dealing with categorical features.
The choice of aggregation function depends on the type of data and the goal of the analysis. For example, sum and mean values can be used to aggregate numerical data.
Binning is a simple technique that groups different values into bins, replacing numerical features with categorical ones. This can be useful for preventing overfitting and increasing the robustness of the machine learning model.
Count encoding is a technique that converts each categorical value to its frequency, replacing each category value with the number of occurrences.
Add Dummy Variables
Most machine learning algorithms can't directly handle categorical features, specifically text values. Therefore, we need to create dummy variables for our categorical features.
Dummy variables are a set of binary (0 or 1) variables that each represent a single class from a categorical feature. This numeric representation allows us to pass the technical requirements for algorithms.
We can create dummy variables using One Hot Encoding, which creates separate columns for each category of the categorical variable. The fit_transform() method from the OneHotEncoder class creates the dummy variables and assigns them binary values.
For example, if we have a categorical variable "Country" with values India, Spain, and Belgium, we can create three separate columns, one for each country. We assign 1 to the column that is true and 0 to the others. This way, we avoid creating an ordinal relationship among the categories.
This process is essential for encoding categorical variables, as it allows us to work with numeric values that can be used to train our model. By creating dummy variables, we can represent categorical features in a way that machine learning algorithms can understand.
Broaden your view: Create Feature for Dataset Huggingface
Split
Data transformation is a crucial step in working with data, and one of the most important techniques is splitting data. Sometimes, data is not connected over rows, but over columns.
In this case, feature splitting comes in handy. Feature splitting is a technique used to extract specific values from a feature, like extracting only the first name from a list of names.
Imagine you have a list of names in one feature, and you want to extract only the first name. You can do this by using the feature splitting technique. It's often used with string data, and it can be a lifesaver in situations like this.
Explore further: First Ai Software Engineer
Machine Learning Techniques
Feature Engineering is crucial in machine learning, and it's all about preparing your data for modeling.
Data cleaning and imputation are essential steps to ensure reliable and consistent information. This involves addressing missing values and inconsistencies in the data.
Feature scaling is another important technique, standardizing the range of numerical features to prevent any single feature from dominating the analysis. This ensures all features contribute equally to the model's training process.
Here are some key techniques to keep in mind:
- Data Cleaning and Imputation
- Feature Scaling
- Feature Encoding (one-hot encoding and label encoding)
- Feature Creation (combining or transforming existing features)
- Feature Selection (selecting the most relevant and informative features)
- Feature Extraction (extracting features from raw data, e.g., dimensionality reduction)
Polynomial
Polynomial features can capture non-linear relationships between variables by creating squared or cubic terms of numerical features. This can be particularly useful in machine learning models that struggle with non-linear data.
Polynomial features create interactions among features, helping to capture relationships among independent variables and potentially decreasing model bias. However, be cautious not to contribute to massive overfitting.
To create polynomial features, you can use the Polynomial Feature module in the sklearn library. This module allows you to specify the degree of interaction and the features you want to cross.
Here are some ways to create interaction features:
- Multiplication or division of two features
- Polynomial combinations
By examining each pair of features and asking yourself if they could be combined in a more useful way, you can create meaningful interaction features. This can involve products, sums, or differences between two features.
Feature-engine is a Python library that extends scikit-learn's transformers to include feature engineering functionalities. It supports a wide range of techniques, including encoding, discretization, and variable transformation.
Machine Learning Techniques and Tools
Feature engineering is a crucial step in the machine learning pipeline, and several tools have been developed to simplify and automate this process. These tools range from libraries that offer specific feature engineering functions to full-fledged automated machine learning (AutoML) platforms that include feature engineering as part of their workflow.
Some of the most popular and effective tools in this space include libraries like Pandas, NumPy, and Scikit-learn, which offer a wide range of feature engineering functions. For example, Pandas can be used for data cleaning and imputation, while NumPy can be used for feature scaling.
Principal Component Analysis (PCA) and Linear Discriminant Analysis (LDA) are two popular dimensionality reduction techniques used in machine learning. PCA reduces the dimensionality of data by projecting it onto principal components that capture the most variance, while LDA is used for classification problems and reduces dimensionality by finding the linear combinations of features that best separate different classes.
For more insights, see: Mlops Tool
Here are some common feature engineering techniques:
- Data cleaning and imputation
- Feature scaling
- Feature encoding (e.g. one-hot encoding, label encoding)
- Feature creation (e.g. creating polynomial features)
- Feature selection (e.g. filter methods, wrapper methods, embedded methods)
- Feature extraction (e.g. PCA, LDA)
These techniques can be used to improve the performance of machine learning models by reducing the impact of irrelevant or redundant features. By applying these techniques, data scientists can create more accurate and reliable models that better capture the underlying patterns and relationships in the data.
You might like: Common Feature Engineering Techniques Ema
Leave One Out Target
Leave One Out Target encoding is a variation of Target encoding that calculates the mean output value for a sample, excluding that sample itself. This is done to avoid information leakage, which would render our tests invalid.
By excluding the sample, Leave One Out Target encoding reduces the risk of overfitting to the training data. This is particularly useful when working with small datasets.
To implement Leave One Out Target encoding, we first define a function that calculates the mean output value for a sample, excluding that sample. This function is then applied to categorical values in the dataset.
Leave One Out Target encoding is built on top of Target encoding, so it inherits its benefits, such as reducing variance in values with few occurrences. By blending the average value with the outcome probability, we can achieve more accurate predictions.
In code, Leave One Out Target encoding looks like this:
This is a key difference from Target encoding, where the mean output value is calculated without excluding the sample.
Automated Feature Engineering
Automated feature engineering is a game-changer for machine learning pipelines. It simplifies the process by automating tasks such as handling missing values, encoding categorical variables, and normalizing/scaling numerical features.
Several tools have been developed to simplify and automate feature engineering, including libraries that offer specific feature engineering functions and full-fledged automated machine learning (AutoML) platforms. AutoML platforms like Auto-sklearn and TPOT automate feature engineering as part of their workflow.
Auto-sklearn, for example, automatically performs feature engineering and model selection, while TPOT uses genetic algorithms to optimize machine learning pipelines, including feature engineering. Featuretools is another open-source library that excels at creating new features from relational datasets using deep feature synthesis (DFS).
See what others are reading: Auto Ml Fit Succeeded
Here are some key features of popular AutoML tools that automate feature engineering:
These tools can save you a significant amount of time and effort, allowing you to focus on more complex tasks in your machine learning pipeline.
Domain Knowledge and Techniques
Domain knowledge is a crucial aspect of feature engineering, and it's amazing how much of a difference it can make in your models. By combining domain expertise with data-driven techniques, you can create features that are tailored to the specific problem you're trying to solve.
Data cleaning and imputation are essential steps in feature engineering, and domain knowledge can help you identify the most important features to focus on. For example, if you're working on a US real-estate model, you might create an indicator variable for transactions during the subprime mortgage housing crisis.
Infusing domain knowledge into your feature engineering process can be a game-changer. It allows you to think creatively and come up with features that are specific to the problem you're trying to solve. For instance, in finance, creating ratios such as price-to-earnings ratio can be a powerful feature.
Check this out: Learning with Errors Problem
To get started with domain knowledge, try to think of specific information you might want to isolate or focus on. Ask yourself questions like "What are the key factors that affect this problem?" or "What are the most important characteristics of the data?" By doing so, you can create features that are both informative and relevant.
Here are some heuristics that can help spark more ideas:
- Best Practices for Feature Engineering
- Python Data Wrangling Tutorial: Cryptocurrency Edition
- Python for Data Science (Ultimate Quickstart Guide)
Remember, domain knowledge is not a one-time thing – it's an ongoing process that requires continuous learning and exploration. By combining domain expertise with data-driven techniques, you can create features that are both informative and relevant, and ultimately improve the performance of your models.
You might like: Tunet and Domain Adaptation
Tools and Libraries
Feature engineering is a crucial step in the machine learning pipeline, and several tools have been developed to simplify and automate this process.
Featuretools is an open-source library for automated feature engineering that excels at creating new features from relational datasets using deep feature synthesis (DFS).
Featuretools automatically creates features from multiple tables and uses DFS to build complex features by stacking primitive operations. It integrates easily with pandas and other data science libraries.
Here are some key features of Featuretools:
- Automatically creates features from multiple tables.
- Uses DFS to build complex features by stacking primitive operations.
- Integrates easily with pandas and other data science libraries.
Python Libraries
Python Libraries can be a game-changer for automating feature engineering.
Featuretools is an open-source library that excels at creating new features from relational datasets using deep feature synthesis (DFS).
Automatically creating features from multiple tables is one of its key features.
Featuretools integrates easily with pandas and other data science libraries.
Here are some benefits of using Featuretools:
H2O.ai
H2O.ai is a powerful tool that provides an open-source platform for scalable machine learning. It's amazing how much it can automate the process of model selection, hyperparameter tuning, and feature engineering.
One of the coolest features of H2O.ai is its automatic feature engineering, which is part of its AutoML workflow. This means you can streamline your machine learning process and save time.
H2O.ai is also super scalable, making it perfect for large datasets. I've seen it handle big data with ease, and it's impressive how quickly it can process it.
If you're looking for a tool that supports a wide range of machine learning algorithms, H2O.ai is a great choice. It's got you covered with its extensive library of algorithms.
Here are some of the key benefits of using H2O.ai:
- Automatic feature engineering as part of the AutoML workflow.
- Scalable to large datasets.
- Supports a wide range of machine learning algorithms.
What Scheme to Use
Choosing the right scheme can be overwhelming, especially with so many options available.
The Scheme vs. Framework debate is a common one, and it largely depends on your project's needs.
If you're building a simple web application, Scheme might be the way to go, as it provides a lightweight and flexible approach.
In contrast, Frameworks like Express.js and Django are better suited for complex projects, offering more structure and organization.
Ultimately, the choice between Scheme and Framework comes down to your project's specific requirements and your personal preference.
For instance, if you're working on a small-scale project, Scheme's minimalism can be a significant advantage, allowing you to focus on your code without unnecessary overhead.
Sources
- https://www.analyticsvidhya.com/blog/2021/10/a-beginners-guide-to-feature-engineering-everything-you-need-to-know/
- https://cgorale111.medium.com/feature-engineering-for-machine-learning-2a20acefcfd8
- https://elitedatascience.com/feature-engineering
- https://rubikscode.net/2021/06/29/top-9-feature-engineering-techniques/
- https://fritz.ai/feature-engineering-in-python/
Featured Images: pexels.com